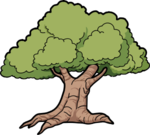 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_ALLOC_SIMPLEGC_H_
22 #define ELM_ALLOC_SIMPLEGC_H_
24 #include <elm/util/BitVector.h>
25 #include <elm/stree/Tree.h>
26 #include <elm/data/List.h>
27 #include <elm/data/BiDiList.h>
28 #include <elm/alloc/DefaultAllocator.h>
47 inline operator T *(
void)
const {
return p; }
51 inline operator bool(
void)
const {
return p; }
67 inline void free(
void *block) { }
74 virtual void endGC(
void);
80 typedef struct block_t {
84 typedef struct chunk_t {
89 List<chunk_t *> chunks;
92 inhstruct::DLList temps;
94 typedef stree::Tree<void *, chunk_t *> tree_t;
97 static inline t::size round(
t::size size) {
return (
size +
sizeof(block_t) - 1) & ~(
sizeof(block_t) - 1); }
Definition: SimpleGC.h:34
T & operator*(void) const
Definition: SimpleGC.h:50
Definition: SimpleGC.h:42
T * operator->(void) const
Definition: SimpleGC.h:49
T * operator&(void) const
Definition: SimpleGC.h:48
void doGC(void)
Definition: alloc_SimpleGC.cpp:121
TempPtr(SimpleGC &gc, T *ptr)
Definition: SimpleGC.h:44
SimpleGC(t::size size=4096)
Definition: alloc_SimpleGC.cpp:84
TempPtr< T > & operator=(T *ptr)
Definition: SimpleGC.h:52
uint64 size
Definition: arch.h:35
virtual void endGC(void)
Definition: alloc_SimpleGC.cpp:231
unsigned char uint8
Definition: arch.h:27
void free(void *block)
Definition: SimpleGC.h:67
Definition: SimpleGC.h:58
virtual void collect(SimpleGC &gc)
Definition: SimpleGC.h:45
virtual ~Temp(void)
Definition: alloc_SimpleGC.cpp:46
virtual void collect(SimpleGC &gc)=0
bool mark(void *data, t::size size)
Definition: alloc_SimpleGC.cpp:182
virtual void collect(void)=0
virtual ~SimpleGC(void)
Definition: alloc_SimpleGC.cpp:105
void clear(void)
Definition: alloc_SimpleGC.cpp:112
Temp(SimpleGC &gc)
Definition: alloc_SimpleGC.cpp:40
void * allocate(t::size size)
Definition: alloc_SimpleGC.cpp:152
virtual void beginGC(void)
Definition: alloc_SimpleGC.cpp:205
uint64 intptr
Definition: arch.h:38