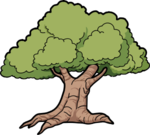 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_SYSTEM_THREAD_H_
22 #define ELM_SYSTEM_THREAD_H_
24 #include <elm/sys/SystemException.h>
25 #include <elm/util/MessageException.h>
27 namespace elm {
namespace sys {
47 virtual void run(
void);
71 virtual void start(
void) = 0;
72 virtual void join(
void) = 0;
73 virtual void kill(
void) = 0;
79 virtual void stop(
void) = 0;
89 virtual void lock(
void) = 0;
90 virtual void unlock(
void) = 0;
Definition: MessageException.h:30
virtual void run(void)
Definition: system_Thread.cpp:54
virtual void kill(void)=0
static Runnable & current(void)
Definition: Thread.h:82
static void setRootRunnable(Runnable &runnable)
void stop(void)
Definition: system_Thread.cpp:75
virtual void start(void)=0
virtual String message(void)
Definition: util_MessageException.cpp:50
Thread * thread(void) const
Definition: Thread.h:49
static Thread * make(Runnable &runnable)
Definition: system_Thread.cpp:427
virtual ~Thread(void)
Definition: system_Thread.cpp:113
virtual bool tryLock(void)=0
Thread(Runnable &runnable)
Definition: system_Thread.cpp:107
virtual void join(void)=0
Runnable & runnable(void) const
Definition: Thread.h:65
virtual void lock(void)=0
Runnable * _runnable
Definition: Thread.h:77
static Thread * current(void)
static Mutex * make(void)
Definition: system_Thread.cpp:443
virtual ~Runnable(void)
Definition: system_Thread.cpp:69
virtual void stop(void)=0
Runnable(void)
Definition: system_Thread.cpp:64
virtual void unlock(void)=0
virtual ~Mutex(void)
Definition: system_Thread.cpp:177
virtual bool isRunning(void)=0
ThreadException(const string &message)
Definition: Thread.h:35