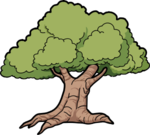 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
22 #ifndef ELM_INHSTRUCT_TREE_H
23 #define ELM_INHSTRUCT_TREE_H
25 #include <elm/PreIterator.h>
26 #include <elm/assert.h>
28 namespace elm {
namespace inhstruct {
34 inline Tree(
void): _children(0), _sibling(0) { }
41 int count(
void)
const;
42 inline bool isEmpty(
void)
const {
return !_children; }
50 inline bool ended (
void)
const {
return !cur; }
53 inline bool equals(
Iter ii)
const {
return cur == ii.cur; }
56 inline operator Tree *()
const {
return item(); }
69 ASSERTP(child,
"null child argument");
70 child->_sibling = _children;
76 Tree *oldSibling = _sibling;
77 newSibling->_sibling = oldSibling;
78 _sibling = newSibling;
82 template <
class TT>
void addAll(
const TT& coll)
89 inline void clear(
void) { _children = 0; }
92 Tree *_children, *_sibling;
97 #endif // ELM_INHSTRUCT_TREE_H
Tree * operator->() const
Definition: Tree.h:58
Tree * operator*() const
Definition: Tree.h:57
Definition: util_WAHVector.cpp:157
bool operator!=(Iter i) const
Definition: Tree.h:62
void removeAll(const TT &coll)
Definition: Tree.h:87
void iter(const C &c, const F &f)
Definition: util.h:95
void removeChild(Tree *child)
Definition: inhstruct_Tree.cpp:145
bool hasChild(Tree *tree) const
Definition: Tree.h:39
Tree * item(void) const
Definition: Tree.h:51
void addSibling(Tree *newSibling)
Definition: Tree.h:75
void next(void)
Definition: Tree.h:52
void prependChild(Tree *child)
Definition: Tree.h:68
void remove(Tree *child)
Definition: Tree.h:85
void appendChild(Tree *child)
Definition: inhstruct_Tree.cpp:115
bool isEmpty(void) const
Definition: Tree.h:42
bool operator==(Iter i) const
Definition: Tree.h:61
bool ended(void) const
Definition: Tree.h:50
Iter & operator++()
Definition: Tree.h:59
int count(void) const
Definition: inhstruct_Tree.cpp:76
void addAll(const TT &coll)
Definition: Tree.h:82
Tree * sibling(void) const
Definition: Tree.h:38
Iter(const Tree *tree)
Definition: Tree.h:48
bool contains(Tree *tree) const
Definition: Tree.h:40
Tree(void)
Definition: Tree.h:34
bool equals(Iter ii) const
Definition: Tree.h:53
Tree * children(void) const
Definition: Tree.h:37
Iter operator++(int)
Definition: Tree.h:60
Iter(const Iter &iter)
Definition: Tree.h:49
void remove(const Iter &iter)
Definition: Tree.h:86
void add(Tree *child)
Definition: Tree.h:81
void clear(void)
Definition: Tree.h:89