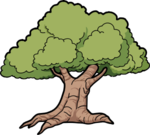 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_SYS_DIRECTORY_H
22 #define ELM_SYS_DIRECTORY_H
24 #include <elm/PreIterator.h>
25 #include <elm/sys/FileItem.h>
27 namespace elm {
namespace sys {
44 bool ended(
void)
const;
50 inline operator t()
const {
return item(); }
70 #endif // ELM_SYS_DIRECTORY_H
Definition: Directory.h:38
void operator++(int)
Definition: Directory.h:54
Iter(LockPtr< Directory > directory)
Definition: system_Directory.cpp:112
t operator*() const
Definition: Directory.h:51
t item(void) const
Definition: system_Directory.cpp:137
t operator->() const
Definition: Directory.h:52
Definition: Directory.h:30
Path & path()
Definition: system_FileItem.cpp:175
LockPtr< FileItem > t
Definition: Directory.h:40
static LockPtr< Directory > make(Path path)
Definition: system_Directory.cpp:47
bool operator!=(const Iter &i) const
Definition: Directory.h:56
Iter & operator++()
Definition: Directory.h:53
bool equals(const Iter &i) const
Definition: system_Directory.cpp:150
~Iter(void)
Definition: system_Directory.cpp:123
Definition: FileItem.h:36
void next(void)
Definition: system_Directory.cpp:145
LockPtr< Directory > toDirectory(void) override
Definition: system_Directory.cpp:76
bool ended(void) const
Definition: system_Directory.cpp:130
bool operator==(const Iter &i) const
Definition: Directory.h:55