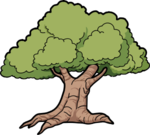 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_INHSTRUCT_DLLIST_H
22 #define ELM_INHSTRUCT_DLLIST_H
24 #include <elm/assert.h>
26 namespace elm {
namespace inhstruct {
36 inline bool atBegin(
void)
const {
return prv == 0; }
37 inline bool atEnd(
void)
const {
return nxt == 0; }
40 ASSERTP(node,
"null node for replacement");
41 nxt->prv = node; node->nxt = nxt;
42 prv->nxt = node; node->prv = prv;
46 ASSERTP(node,
"null node to insert");
47 nxt->prv = node; node->nxt = nxt;
48 nxt = node; node->prv =
this;
52 ASSERTP(node,
"null node to insert");
53 prv->nxt = node; node->prv = prv;
54 prv = node; node->nxt =
this;
58 { prv->nxt = nxt; nxt->prv = prv; }
60 { ASSERTP(!nxt->
atEnd(),
"no next node"); nxt->
remove(); }
62 { ASSERTP(!prv->
atBegin(),
"no previous node"); prv->
remove(); }
71 hd.nxt = &tl; hd.prv = 0;
72 tl.prv = &hd; tl.nxt = 0;
95 inline bool isEmpty(
void)
const {
return hd.nxt == &tl; }
101 for(
DLNode *cur = hd.nxt; cur != &tl; cur =cur->nxt)
107 { ASSERTP(node,
"null node added"); hd.
insertAfter(node); }
109 { ASSERTP(node,
"null node added"); tl.
insertBefore(node); }
119 #endif // ELM_INHSTRUCT_DLLIST_H
DLNode * first(void) const
Definition: DLList.h:93
DLNode * next(void) const
Definition: DLList.h:34
bool atEnd(void) const
Definition: DLList.h:37
DLNode * head(void) const
Definition: DLList.h:96
void removeNext(void)
Definition: DLList.h:59
void addFirst(DLNode *node)
Definition: DLList.h:106
int count(void) const
Definition: DLList.h:99
DLNode * previous(void) const
Definition: DLList.h:35
DLNode * tail(void) const
Definition: DLList.h:97
void removeFirst(void)
Definition: DLList.h:110
bool atBegin(void) const
Definition: DLList.h:36
void insertAfter(DLNode *node)
Definition: DLList.h:45
void replace(DLNode *node)
Definition: DLList.h:39
DLList(DLList &list)
Definition: DLList.h:75
ListPrinter< T > list(const T &l, cstring s="", typename ListPrinter< T >::fun_t f=ListPrinter< T >::asis)
Definition: Output.h:321
void remove(void)
Definition: DLList.h:57
void addLast(DLNode *node)
Definition: DLList.h:108
DLList(void)
Definition: DLList.h:70
bool isEmpty(void) const
Definition: DLList.h:95
DLNode * last(void) const
Definition: DLList.h:94
void insertBefore(DLNode *node)
Definition: DLList.h:51
void removeLast(void)
Definition: DLList.h:112
void removePrevious(void)
Definition: DLList.h:61