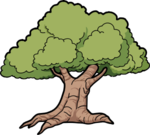 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_DATA_SORTEDLIST_H_
22 #define ELM_DATA_SORTEDLIST_H_
27 #include <elm/compare.h>
28 #include <elm/util/Option.h>
33 template <
class T,
class C = Comparator<T>,
class A = DefaultAlloc >
55 {
for(
const auto i: c)
if(!
contains(i))
return false;
return true; }
65 inline Iter
items(
void)
const {
return Iter(*
this); }
67 inline operator Iter(
void)
const {
return items(); }
69 inline Iter
end(
void)
const {
return Iter(); }
73 for(; i() && j(); i++, j++)
86 for(
typename list_t::PrecIter current(
list); current(); current++)
94 template <
class CC>
inline void addAll (
const CC &c)
97 template <
class CC>
inline void removeAll(
const CC &c)
107 Iter i =
iter;
for(; i(); i++) {
109 if(cmp > 0)
continue;
else if(!cmp)
return i;
else return end();
115 inline Iter
nth(
int n)
const { Iter i(*
this);
while(n != 0 && i()) { n--; i++; };
return i; }
T & at(const Iter &i)
Definition: SortedList.h:114
void clear(void)
Definition: List.h:129
Iter(void)
Definition: SortedList.h:62
Definition: util_WAHVector.cpp:157
void iter(const C &c, const F &f)
Definition: util.h:95
void addLast(const T &value)
Definition: List.h:158
bool contains(const T &item) const
Definition: List.h:120
List< T, CompareEquiv< C >, A > list_t
Definition: SortedList.h:36
Iter items(void) const
Definition: SortedList.h:65
bool isEmpty(void) const
Definition: SortedList.h:57
Iter find(const T &item) const
Definition: SortedList.h:113
void add(const T &value)
Definition: SortedList.h:85
bool contains(const T &item) const
Definition: SortedList.h:53
Definition: SortedList.h:34
bool operator&(const T &e) const
Definition: SortedList.h:119
void addAll(const CC &c)
Definition: SortedList.h:94
void removeLast(void)
Definition: SortedList.h:48
Iter end(void) const
Definition: SortedList.h:69
T & last(void)
Definition: List.h:147
list_t list
Definition: SortedList.h:126
void removeAll(const C &items)
Definition: List.h:134
bool operator==(const SortedList< T > &l) const
Definition: SortedList.h:78
const T & operator[](int k) const
Definition: SortedList.h:121
void copy(const SortedList< T > &l)
Definition: SortedList.h:83
void remove(const T &item)
Definition: SortedList.h:96
const C & comparator() const
Definition: SortedList.h:44
T t
Definition: SortedList.h:38
Iter find(const T &item, const Iter &iter) const
Definition: SortedList.h:106
E & equivalence()
Definition: List.h:54
void clear(void)
Definition: SortedList.h:82
bool operator!=(const SortedList< T > &l) const
Definition: SortedList.h:79
Iter(const self_t &_list)
Definition: SortedList.h:63
const T & last(void) const
Definition: SortedList.h:105
void copy(const List< T, E, A > &list)
Definition: List.h:58
SortedList< T, C, A > self_t
Definition: SortedList.h:39
SortedList< T > & operator+=(const T &v)
Definition: SortedList.h:100
A & allocator()
Definition: List.h:56
int count(void) const
Definition: SortedList.h:51
bool equals(const SortedList< T > &l) const
Definition: SortedList.h:71
int count(void) const
Definition: List.h:119
Iter nth(int n) const
Definition: SortedList.h:115
A & allocator()
Definition: SortedList.h:45
void removeFirst(void)
Definition: List.h:164
bool isEmpty(void) const
Definition: List.h:122
void set(const Iter &pos, const T &item)
Definition: List.h:166
void removeLast(void)
Definition: List.h:165
void removeFirst(void)
Definition: SortedList.h:47
void remove(const Iter &iter)
Definition: SortedList.h:99
T & operator[](int k)
Definition: SortedList.h:120
void remove(const T &value)
Definition: List.h:136
void addBefore(PrecIter &pos, const T &value)
Definition: List.h:161
const T & at(const Iter &i) const
Definition: List.h:126
bool containsAll(const CC &c) const
Definition: SortedList.h:54
T & first(void)
Definition: List.h:145
void set(Iter i, const T &val)
Definition: SortedList.h:125
void removeAll(const CC &c)
Definition: SortedList.h:97
SortedList< T > & operator-=(const T &v)
Definition: SortedList.h:101
Iter operator*(void) const
Definition: SortedList.h:66
const T & first(void) const
Definition: SortedList.h:104
void addAll(const C &items)
Definition: List.h:132
SortedList< T, C > & operator=(const SortedList< T, C > &sl)
Definition: SortedList.h:118
SortedList(void)
Definition: SortedList.h:41
Definition: SortedList.h:60
Iter begin(void) const
Definition: SortedList.h:68
C & comparator()
Definition: SortedList.h:43
SortedList(const SortedList< T, C, A > &l)
Definition: SortedList.h:42