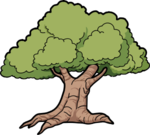 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_DATA_ARRAYLIST_H_
22 #define ELM_DATA_ARRAYLIST_H_
24 #include <elm/assert.h>
25 #include <elm/PreIterator.h>
26 #include <elm/equiv.h>
30 template <
class T,
class E = Equiv<T> >
34 typedef struct chunk_t {
41 inline ArrayList(
int n = 6): _csize(1 << n), _size(0), _avail(0), _fst(0), _lst(0) { }
49 { ASSERT(start <= a._size); c = a.chunk(start); i = start; }
51 : a(
array), i(end ? a._size : 0), c(end ? a._lst : a._fst) { }
52 inline bool ended(
void)
const {
return i < a._size; }
53 inline const T&
item(
void)
const {
return c->items[a.offset(i)]; }
54 inline void next(
void) { i++;
if(!
offset(i)) c = c->next; }
55 inline int index(
void)
const {
return i; }
63 inline int count(
void)
const {
return _size; }
65 {
for(
Iter i(*
this); i; i++)
if(
E::equals(item, *i))
return true;
return false; }
66 template<
template<
class _ >
class C>
68 {
for(
typename C<T>::iter i(coll); i; i++)
if(!
contains(*i))
return false;
return true; }
69 inline bool isEmpty(
void)
const {
return !_fst; }
74 {
for(chunk_t *c = _fst, *n = 0; c !=
nullptr; c = n) { n = c->next;
delete n; }
75 _size = _avail = 0; _fst = _lst = 0; }
76 inline void add(
const T& v)
77 {
if(_size == _avail) extend(); _lst->items[
offset(_size)] = v; _size++; }
78 template <
template <
class _>
class C>
82 template <
template <
class _>
class C>
89 inline int length(
void)
const {
return _size; }
90 inline const T &
get(
int index)
const
91 { ASSERT(index < _size);
return chunk(index)->items[
offset(index)]; }
93 {
for(
Iter i(*
this, start); i; i++)
if(
E::equals(*i,
value))
return i.index();
return -1; }
95 {
int r = -1;
for(
Iter i(*
this, start); i && i.
index() <= start; i++)
if(
E::equals(*i,
value)) r = i.index();
return r; }
100 { _lst = chunk(
length);
for(chunk_t *c = _lst, *n; c; c = n) { n = c->next;
delete c; } _size =
length; }
101 inline void set(
int index,
const T &item) { chunk(index)->items[
offset(index)] = item; }
103 inline T &
get(
int index) {
return chunk(index)->items[
offset(index)]; }
113 inline const T &
first(
void)
const { ASSERT(_fst);
return _fst->items[0]; }
114 inline const T &
last(
void)
const { ASSERT(_lst);
return _lst->items[
offset(_size) - 1]; }
117 {
while(start && !
E::equals(item, *start)) start++;
return start; }
130 { chunk_t *c =
new chunk_t; c->next = 0;
if(_lst) _lst->next = c;
else _fst = c; _lst = c; _avail += _csize; }
131 inline int offset(
int n)
const {
return n & (_csize - 1); }
132 inline chunk_t *chunk(
int n) { chunk_t *c = _fst;
for(; n >= _csize; n -= _csize) c = c->next();
return c; }
134 int _csize, _size, _avail;
135 chunk_t *_fst, *_lst;
Iter(const ArrayList< T > &array, bool end)
Definition: ArrayList.h:50
Definition: util_WAHVector.cpp:157
void removeAt(const Iter &it)
Definition: ArrayList.h:110
void iter(const C &c, const F &f)
Definition: util.h:95
void removeAll(const C< T > &items)
Definition: ArrayList.h:83
void addAll(const C< T > &items)
Definition: ArrayList.h:79
void insert(const Iter &it, T item)
Definition: ArrayList.h:106
bool contains(const T &item)
Definition: ArrayList.h:64
void addAfter(const Iter &pos, const T &item)
Definition: ArrayList.h:124
bool equals(const C1 &c1, const C2 &c2)
Definition: util.h:107
int length(void) const
Definition: ArrayList.h:89
void removeLast(void)
Definition: ArrayList.h:123
Iter(const ArrayList< T > &array, int start)
Definition: ArrayList.h:48
void clear(void)
Definition: ArrayList.h:73
Definition: ArrayList.h:31
T & get(int index)
Definition: ArrayList.h:103
void set(int index, const T &item)
Definition: ArrayList.h:101
const T & operator[](int index) const
Definition: ArrayList.h:96
void remove(Iter i)
Definition: ArrayList.h:84
int indexOf(const T &value, int start=0) const
Definition: ArrayList.h:92
Iter(const ArrayList< T > &array)
Definition: ArrayList.h:47
bool ended(void) const
Definition: ArrayList.h:52
const T & get(int index) const
Definition: ArrayList.h:90
bool containsAll(const C< T > &coll)
Definition: ArrayList.h:67
const T & item(void) const
Definition: ArrayList.h:53
void addBefore(const Iter &pos, const T &item)
Definition: ArrayList.h:125
Definition: ArrayList.h:44
int count(void) const
Definition: ArrayList.h:63
void next(void)
Definition: ArrayList.h:54
void remove(const T &item)
Definition: ArrayList.h:80
ArrayList(int n=6)
Definition: ArrayList.h:41
const T & first(void) const
Definition: ArrayList.h:113
Iter find(const T &item)
Definition: ArrayList.h:115
void shrink(int length)
Definition: ArrayList.h:99
~ArrayList(void)
Definition: ArrayList.h:42
void insert(int index, const T &item)
Definition: ArrayList.h:105
T & operator[](int index)
Definition: ArrayList.h:104
void addLast(const T &item)
Definition: ArrayList.h:121
void addFirst(const T &item)
Definition: ArrayList.h:120
bool isEmpty(void) const
Definition: ArrayList.h:69
Iter find(const T &item, Iter start) const
Definition: ArrayList.h:116
int index(void) const
Definition: ArrayList.h:55
friend class iter
Definition: ArrayList.h:32
const T & last(void) const
Definition: ArrayList.h:114
void add(const T &v)
Definition: ArrayList.h:76
void removeAt(int index)
Definition: ArrayList.h:109
void set(const Iter &it, const T &item)
Definition: ArrayList.h:102
void removeFirst(void)
Definition: ArrayList.h:122
uint64 offset
Definition: arch.h:36
int lastIndexOf(const T &value, int start=-1) const
Definition: ArrayList.h:94