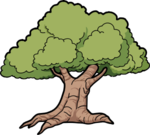 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
22 #ifndef ELM_DYNDATA_ABSTRACTCOLLECTION_H
23 #define ELM_DYNDATA_ABSTRACTCOLLECTION_H
25 #include <elm/PreIterator.h>
28 namespace elm {
namespace dyndata {
35 virtual bool ended(
void)
const = 0;
36 virtual T
item(
void)
const = 0;
37 virtual void next(
void) = 0;
48 inline bool ended(
void)
const {
return i->ended(); }
49 inline T
item(
void)
const {
return i->item(); }
50 inline void next(
void) {
i->next(); }
61 virtual int count(
void) = 0;
62 virtual bool contains(
const T& item)
const = 0;
63 virtual bool isEmpty(
void)
const = 0;
74 virtual void clear(
void) = 0;
75 virtual void add(
const T& item) = 0;
77 virtual void remove(
const T& item) = 0;
84 #endif // ELM_DYNDATA_ABSTRACTCOLLECTION_H
virtual void next(void)=0
Iter(AbstractIter< T > *iter)
Definition: AbstractCollection.h:45
Definition: util_WAHVector.cpp:157
Definition: AbstractCollection.h:32
virtual void add(const T &item)=0
T item(void) const
Definition: AbstractCollection.h:49
virtual bool ended(void) const =0
Definition: AbstractCollection.h:58
virtual ~AbstractIter(void)
Definition: AbstractCollection.h:34
virtual Iter< T > items(void) const =0
virtual bool contains(const T &item) const =0
virtual ~AbstractCollection(void)
Definition: AbstractCollection.h:60
virtual ~MutableAbstractCollection(void)
Definition: AbstractCollection.h:73
Definition: AbstractCollection.h:43
LockPtr< AbstractIter< T > > i
Definition: AbstractCollection.h:53
Iter< T > & operator=(const Iter< T > &iter)
Definition: AbstractCollection.h:51
virtual void removeAll(const AbstractCollection< T > &items)=0
virtual T item(void) const =0
virtual int count(void)=0
Definition: AbstractCollection.h:71
AbstractIter< T > * instance(void) const
Definition: AbstractCollection.h:47
virtual bool isEmpty(void) const =0
Iter< T > operator*(void) const
Definition: AbstractCollection.h:66
bool ended(void) const
Definition: AbstractCollection.h:48
virtual void clear(void)=0
virtual void remove(const T &item)=0
void next(void)
Definition: AbstractCollection.h:50
Iter(const Iter< T > &iter)
Definition: AbstractCollection.h:46
virtual void addAll(const AbstractCollection< T > &items)=0