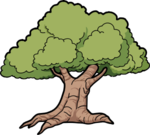 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_JSON_PARSER_H_
22 #define ELM_JSON_PARSER_H_
26 #include <elm/sys/Path.h>
28 namespace elm {
namespace json {
37 virtual void onField(
string name);
81 void error(
string message);
84 void pushBack(
char c);
virtual void endObject(void)
Definition: json_Parser.cpp:60
virtual void onValue(bool value)
Definition: json_Parser.cpp:103
void parse(string s)
Definition: json_Parser.cpp:164
virtual void onNull(void)
Definition: json_Parser.cpp:93
IntFormat base(int base, IntFormat fmt)
Definition: Output.h:256
virtual void onField(string name)
Definition: json_Parser.cpp:85
Parser(Maker &maker)
Definition: json_Parser.cpp:156
virtual void endArray(void)
Definition: json_Parser.cpp:75
typename type_info< T >::in_t in
Definition: type_info.h:283
void parse(const char *s)
Definition: Parser.h:50
virtual void beginArray(void)
Definition: json_Parser.cpp:67
virtual ~Maker(void)
Definition: json_Parser.cpp:45
void parse(cstring s)
Definition: Parser.h:49
virtual void beginObject(void)
Definition: json_Parser.cpp:52
Definition: InStream.h:29