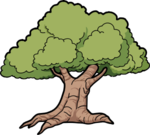 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_DATA_HASHSET_H_
22 #define ELM_DATA_HASHSET_H_
25 #include "HashTable.h"
26 #include <elm/adapter.h>
30 template <
class T,
class H = HashKey<T>,
class A = DefaultAlloc>
38 inline const H&
hash()
const {
return _tab.
hash(); }
47 {
for(
typename C::Iter i(coll); i(); i++)
if(!
contains(*i))
return false;
return true; }
56 inline bool ended(
void)
const {
return i.ended(); }
57 inline const T&
item(
void)
const {
return i.
item(); }
58 inline void next(
void) { i.next(); }
59 inline bool equals(
const Iter& it)
const {
return i.equals(it.i); }
67 {
return _tab.
equals(s._tab); }
74 template <
class C>
void addAll(
const C& coll)
75 {
for(
typename C::Iter i(coll); i(); i++)
add(*i); }
78 {
for(
const auto x: c)
remove(x); }
88 {
for(
const auto x: *
this)
if(!s.
contains(x))
return false;
return true; }
94 {
for(
const auto x: c)
insert(x); }
96 {
for(
const auto x: c)
remove(x); }
99 for(
const auto x: *
this)
124 int minEntry(
void)
const {
return _tab.minEntry(); }
125 int maxEntry(
void)
const {
return _tab.maxEntry(); }
126 int zeroEntry(
void)
const {
return _tab.zeroEntry(); }
127 int size(
void)
const {
return _tab.size(); }
138 template <
class T,
class H,
class A>
void insert(const T &val)
Definition: HashSet.h:86
HashSet(const HashSet< T > &s)
Definition: HashSet.h:37
void add(const T &value)
Definition: List.h:131
self_t & operator+=(const HashSet< T > &s)
Definition: HashSet.h:105
bool operator<=(const HashSet< T > &s) const
Definition: HashSet.h:89
self_t operator&(const HashSet< T > &s) const
Definition: HashSet.h:116
self_t operator+=(const T &x)
Definition: HashSet.h:82
Iter operator*(void) const
Definition: HashSet.h:132
static const self_t null
Definition: HashSet.h:121
H & hash()
Definition: HashSet.h:39
void removeAll(const C &c)
Definition: HashSet.h:77
bool operator==(const HashSet< T > &s) const
Definition: HashSet.h:68
self_t operator+(const HashSet< T > &s) const
Definition: HashSet.h:110
int count(void) const
Definition: HashSet.h:44
self_t & operator*=(const HashSet< T > &s)
Definition: HashSet.h:109
bool isEmpty(void) const
Definition: HashSet.h:48
self_t operator*(const HashSet< T > &s) const
Definition: HashSet.h:118
const H & hash() const
Definition: HashTable.h:98
const T & item(void) const
Definition: HashSet.h:57
bool ended(void) const
Definition: HashSet.h:56
Definition: HashTable.h:135
bool equals(const Iter &it) const
Definition: HashSet.h:59
bool contains(const T &val) const
Definition: HashSet.h:45
const A & allocator() const
Definition: HashTable.h:100
bool operator<(const HashSet< T > &s) const
Definition: HashSet.h:91
void put(const T &data)
Definition: HashTable.h:114
self_t operator-=(const T &x)
Definition: HashSet.h:83
Iter(const HashSet &set, bool end)
Definition: HashSet.h:55
void clear(void)
Definition: HashSet.h:72
void add(const T &val)
Definition: HashSet.h:73
const T & item(void) const
Definition: HashTable.h:139
bool equals(const HashTable< T > &h) const
Definition: HashTable.h:144
Iter end(void) const
Definition: HashSet.h:64
bool operator!=(const HashSet< T > &s) const
Definition: HashSet.h:69
void copy(const HashTable< T, H > &t)
Definition: HashTable.h:194
void addAll(const C &coll)
Definition: HashSet.h:74
bool hasKey(const T &key) const
Definition: HashTable.h:107
void meet(const HashSet< T > &c)
Definition: HashSet.h:97
const H & hash() const
Definition: HashSet.h:38
uint64 size
Definition: arch.h:35
HashSet< T, H, A > self_t
Definition: HashSet.h:34
void diff(const HashSet< T > &c)
Definition: HashSet.h:95
bool subsetOf(const HashSet< T > &s) const
Definition: HashSet.h:87
void join(const HashSet< T > &c)
Definition: HashSet.h:93
self_t operator-(const HashSet< T > &s) const
Definition: HashSet.h:114
HashSet(int size=211)
Definition: HashSet.h:36
self_t & operator-=(const HashSet< T > &s)
Definition: HashSet.h:107
int count(void) const
Definition: HashTable.h:124
void clear(void)
Definition: HashTable.h:152
void remove(const T &key)
Definition: HashTable.h:166
Iter items(void) const
Definition: HashSet.h:131
Definition: HashTable.h:32
bool equals(const HashSet< T > &s) const
Definition: HashSet.h:66
void remove(const Iter &i)
Definition: HashSet.h:79
bool operator>=(const HashSet< T > &s) const
Definition: HashSet.h:90
A & allocator()
Definition: HashSet.h:41
bool containsAll(const C &coll)
Definition: HashSet.h:46
self_t & operator&=(const HashSet< T > &s)
Definition: HashSet.h:108
Iter(const HashSet &set)
Definition: HashSet.h:54
self_t operator=(const HashSet< T > &s)
Definition: HashSet.h:81
void copy(const HashSet< T > &s)
Definition: HashSet.h:80
void remove(const T &val)
Definition: HashSet.h:76
Iter begin(void) const
Definition: HashSet.h:63
const A & allocator() const
Definition: HashSet.h:40
void next(void)
Definition: HashSet.h:58
bool operator>(const HashSet< T > &s) const
Definition: HashSet.h:92
self_t operator|(const HashSet< T > &s) const
Definition: HashSet.h:112
self_t & operator|=(const HashSet< T > &s)
Definition: HashSet.h:106
bool isEmpty(void) const
Definition: HashTable.h:121