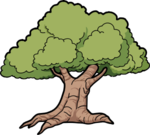 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_DATA_BAG_H_
22 #define ELM_DATA_BAG_H_
24 #include <elm/data/Array.h>
25 #include <elm/data/Vector.h>
33 static inline void operator delete(
void *
p)
34 {
delete []
reinterpret_cast<char *
>(
p); }
45 inline int count()
const {
return cnt; }
46 inline bool isEmpty()
const {
return cnt == 0; }
48 inline const T&
get(
int i)
const { ASSERTP(0 <= i && i < cnt,
"bad index");
return *(base() + i); }
49 inline T&
get(
int i) { ASSERTP(0 <= i && i < cnt,
"bad index");
return *(base() + i); }
56 inline Iter(
const Bag<T> *bag,
int idx = 0): b(*bag), i(idx) { }
57 inline bool ended()
const {
return i >= b->count(); }
58 inline const T&
item()
const {
return b[i]; }
59 inline void next() { i++; }
60 inline bool equals(
const Iter& ii)
const {
return &b == &ii.b && i == ii.i; }
70 {
for(
auto y: *
this)
if(x == y)
return true;
return false; }
72 {
for(
auto x: c)
if(!
contains(x))
return false;
return true; }
75 if(cnt != c.
count())
return false;
76 for(
int i = 0; i < cnt; i++)
if(
get(i) != c.
get(i))
return false;
83 inline int indexOf(
const T& x,
int i = -1)
const
84 {
for(i++; i < cnt; i++)
if(
get(i) == x)
return i;
return -1; }
86 {
if(i < 0) i = cnt; i--;
while(i >= 0 && x !=
get(i)) i--;
return i; }
89 static inline void *
operator new(std::size_t
size,
int cnt)
90 {
return new char[
size +
sizeof(T) * cnt]; }
93 inline const T *base()
const {
return reinterpret_cast<const T *
>(
this + 1); }
94 inline T *base() {
return reinterpret_cast<T *
>(
this + 1); }
const T & item() const
Definition: Bag.h:58
bool equals(const Bag< T > &c) const
Definition: Bag.h:74
static Bag< T > * make(const Array< T > &a)
Definition: Bag.h:39
const T * buffer(void) const
Definition: Array.h:40
void copy(T *target, const T *source, int size)
Definition: array.h:70
const T & operator[](int i) const
Definition: Bag.h:51
Iter begin() const
Definition: Bag.h:66
const T & get(int i) const
Definition: Bag.h:48
Array< const T > asArray(void) const
Definition: Vector.h:53
Printable< T, M > p(const T &data, const M &man)
Definition: Output.h:302
int count() const
Definition: Bag.h:45
bool isEmpty() const
Definition: Bag.h:46
bool operator==(const Bag< T > &b) const
Definition: Bag.h:79
bool containsAll(const Bag< T > &c) const
Definition: Bag.h:71
T & get(int i)
Definition: Bag.h:49
bool contains(const T &x) const
Definition: Bag.h:69
Iter end() const
Definition: Bag.h:67
bool equals(const Iter &ii) const
Definition: Bag.h:60
Iter(const Bag< T > *bag, int idx=0)
Definition: Bag.h:56
int lastIndexOf(const T &x, int i=-1) const
Definition: Bag.h:85
uint64 size
Definition: arch.h:35
bool operator!=(const Bag< T > &b) const
Definition: Bag.h:80
void next()
Definition: Bag.h:59
bool ended() const
Definition: Bag.h:57
T & operator[](int i)
Definition: Bag.h:52
int indexOf(const T &x, int i=-1) const
Definition: Bag.h:83
int count(void) const
Definition: Array.h:71
static Bag< T > * make(int count, const T *items)
Definition: Bag.h:36
static Bag * make(const Vector< T > &v)
Definition: Bag.h:42
int length() const
Definition: Bag.h:82