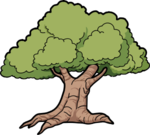 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_SYS_FILE_ITEM_H
22 #define ELM_SYS_FILE_ITEM_H
25 #include <elm/util/LockPtr.h>
26 #include <elm/sys/Path.h>
27 #include <elm/sys/SystemException.h>
29 namespace elm {
namespace sys {
59 #endif // ELM_SYS_FILE_ITEM_H
bool isReadable()
Definition: system_FileItem.cpp:184
Directory * parent
Definition: FileItem.h:50
FileItem(Path path, ino_t inode)
Definition: system_FileItem.cpp:76
bool isDeletable()
Definition: system_FileItem.cpp:212
bool isWritable()
Definition: system_FileItem.cpp:198
String name()
Definition: system_FileItem.cpp:166
virtual ~FileItem()
Definition: system_FileItem.cpp:82
Definition: Directory.h:30
Path & path()
Definition: system_FileItem.cpp:175
virtual LockPtr< Directory > toDirectory()
Definition: system_FileItem.cpp:157
static LockPtr< FileItem > get(Path path)
Definition: system_FileItem.cpp:97
Path _path
Definition: FileItem.h:51
virtual LockPtr< File > toFile()
Definition: system_FileItem.cpp:148
Definition: FileItem.h:36
ino_t ino
Definition: FileItem.h:52