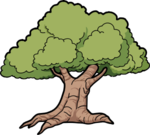 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_XOM_STRING_H
22 #define ELM_XOM_STRING_H
24 #include <elm/string.h>
27 namespace elm {
namespace xom {
63 #endif // ELM_XOM_STRING_H
String(const CString &str)
Definition: String.h:42
void escape(io::Output &out) const
Definition: String.h:51
io::Output & operator<<(io::Output &out, String str)
Definition: String.h:58
typename type_info< T >::out_t out
Definition: type_info.h:284
Definition: StringBuffer.h:18
elm::string escape(void) const
Definition: String.h:52
unsigned char char_t
Definition: String.h:32
void free(void)
Definition: xom_String.cpp:45
const char * buf
Definition: CString.h:19
CString cstring
Definition: CString.h:62
String & operator=(const String &str)
Definition: String.h:55
String(const char *str)
Definition: String.h:40
String(const String &str)
Definition: String.h:43
void copy(void)
Definition: xom_String.cpp:37
Definition: OutStream.h:30
string str(const char *s)
Definition: String.h:150
String(void)
Definition: String.h:39
String(const char_t *str)
Definition: String.h:41