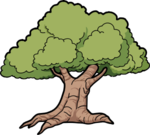 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_ALLOC_ABSTRACTGC_H_
22 #define ELM_ALLOC_ABSTRACTGC_H_
24 #include <elm/data/List.h>
25 #include <elm/types.h>
35 virtual void clean(
void *
p);
45 virtual void free(
void *block);
49 virtual void runGC() = 0;
53 virtual void clean() = 0;
Printable< T, M > p(const T &data, const M &man)
Definition: Output.h:302
Definition: AbstractGC.h:31
virtual ~AbstractGC()
Definition: alloc_AbstractGC.cpp:90
Definition: AbstractGC.h:38
virtual void clean(void *p)
Definition: alloc_AbstractGC.cpp:54
virtual bool mark(void *data, t::size size)=0
virtual void * allocate(t::size size)=0
virtual void collect(AbstractGC &gc)=0
virtual void free(void *block)
Definition: alloc_AbstractGC.cpp:125
GCManager & manager
Definition: AbstractGC.h:56
uint64 size
Definition: arch.h:35
virtual ~GCManager()
Definition: alloc_AbstractGC.cpp:37
AbstractGC(GCManager &m)
Definition: AbstractGC.h:40
void * alloc()
Definition: AbstractGC.h:46