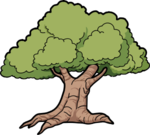 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_RTTI_ENUM_H_
22 #define ELM_RTTI_ENUM_H_
24 #include <elm/data/Vector.h>
27 namespace elm {
namespace rtti {
37 inline Value(
void): _value(0) { }
40 inline int value(
void)
const {
return _value; }
65 virtual const Type&
type(
void)
const;
66 virtual int valueFor(
string text)
const;
71 virtual bool isEnum(
void)
const;
83 #define ELM_DECLARE_ENUM(name) \
84 namespace elm { namespace rtti { template <> const elm::rtti::Type& _type<name>::_(void); } } \
85 inline elm::io::Output& operator<<(elm::io::Output& out, name value) { out << elm::type_of<name>().asEnum().nameFor(value); return out; } \
86 inline elm::io::Input& operator>>(elm::io::Input& in, name& value) \
87 { value = static_cast<name>(elm::type_of<name>().asEnum().valueFor(in.scanWord())); return in; }
89 #define ELM_DEFINE_ENUM(type, desc) \
90 namespace elm { namespace rtti { template <> const elm::rtti::Type& _type<type>::_(void) { return desc; } } }
92 #define ELM_BEGIN_ENUM(type) \
93 namespace elm { namespace rtti { template <> const elm::rtti::Type& _type<type>::_(void) { \
94 static elm::rtti::Enum _(Enum::make(#type)
96 #define ELM_END_ENUM \
102 #ifndef ELM_NO_SHORTCUT
103 # define DECLARE_ENUM(name) ELM_DECLARE_ENUM(name)
104 # define DEFINE_ENUM(type, desc) ELM_DEFINE_ENUM (type, desc)
105 # define BEGIN_ENUM(type) ELM_BEGIN_ENUM(type)
106 # define END_ENUM ELM_END_ENUM
make & alias(cstring name, int value)
Definition: Enum.h:51
Value(void)
Definition: Enum.h:37
virtual int valueFor(string text) const
Definition: rtti.cpp:1218
string name(void) const
Definition: Type.h:91
virtual const Enumerable & asEnum(void) const
Definition: rtti.cpp:1255
Vector< Value >::Iter Iter
Definition: Enum.h:61
Iter values(void) const
Definition: Enum.h:62
virtual bool isEnum(void) const
Definition: rtti.cpp:1249
Enum(const make &make)
Definition: rtti.cpp:1191
rtti::Enum::Value value(cstring name, int value)
Definition: Enum.h:79
make(cstring name)
Definition: Enum.h:49
virtual bool canCast(const Type *t) const
Definition: rtti.cpp:1243
make & value(cstring name, int value)
Definition: Enum.h:50
virtual const Type & type(void) const
Definition: rtti.cpp:1210
static Value end(void)
Definition: Enum.h:36
Value(cstring name, int value)
Definition: Enum.h:38
cstring name(void) const
Definition: Enum.h:39
int value(void) const
Definition: Enum.h:40
virtual cstring nameFor(int value) const
Definition: rtti.cpp:1227