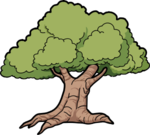 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_IO_INPUT_H
22 #define ELM_IO_INPUT_H
24 #include <elm/enum_info.h>
26 #include <elm/types.h>
27 #include <elm/string/String.h>
28 #include <elm/string/CString.h>
29 #include <elm/io/InStream.h>
31 namespace elm {
namespace io {
40 inline bool ended()
const {
return state & ENDED; }
41 inline bool failed()
const {
return state & FAILED; }
42 inline bool error()
const {
return state & IO_ERROR; }
43 inline bool ok()
const {
return state == 0; }
44 inline void resetState() { state &= ~(FAILED | IO_ERROR); }
92 inline bool ended()
const {
return e; }
93 inline string item()
const {
return l; }
95 inline bool equals(
const LineIter& i)
const {
return &in == &i.in && e == i.e; }
114 [[noreturn]]
static void unsupported(
void);
129 #endif // ELM_IO_INPUT_H
int int32
Definition: arch.h:30
unsigned short uint16
Definition: arch.h:29
IntFormat base(int base, IntFormat fmt)
Definition: Output.h:256
long int64
Definition: arch.h:32
CString cstring
Definition: CString.h:62
short int16
Definition: arch.h:28
static T fromString(const string &name)
Definition: enum_info.h:47
unsigned long uint64
Definition: arch.h:33
unsigned int uint32
Definition: arch.h:31
string str(const char *s)
Definition: String.h:150
sys::SystemInStream & in
Definition: system_SystemIO.cpp:116
Definition: InStream.h:29