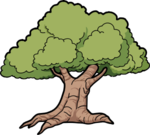 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
23 #include "../include/elm/equiv.h"
25 namespace elm {
namespace concept {
260 template <
template <
class _>
class C>
372 void add(
const T& item);
384 void remove(
const T& item);
451 void insert(
const T& item);
588 const T&
get(
int index)
const;
639 void set(
int index,
const T& item);
690 void insert(
int index,
const T& item);
742 const T&
top(
void)
const;
761 void push(
const T& item);
797 const T&
head(
void)
const;
809 void put(
const T& item);
854 static bool equals(
const T& object1,
const T& object2);
870 bool isEqual(
const T& object1,
const T& object2);
904 static int compare(
const T& object1,
const T& object2);
914 int doCompare(
const T& object1,
const T& object2);
941 static bool equals(
const T& val1,
const T& val2);
950 static bool isEqual(
const T& val1,
const T& val2);
989 static bool equals(
const T& v1,
const T& v2);
1005 static bool lessThan(
const T& v1,
const T& v2);
1013 static int compare(
const T& v1,
const T& v2);
1026 template <
class K,
class T>
1043 const T&
get(
const K& key,
const T& def)
const;
1050 bool hasKey(
const K& key)
const;
1120 template <
class K,
class T>
1134 void put(
const K& key,
const T&
value);
1177 const T&
first(
void)
const;
1183 const T&
last(
void)
const;
1361 template <
class K,
class T>
1375 static const K&
key(
const T&
value);
1411 bool test(
const T& item);
Definition: concepts.h:836
virtual void * chunkFilled(t::size size)
Definition: alloc_StackAllocator.cpp:92
MutableCollection< T > & operator-=(const T &item)
Set< T > operator+=(const Set< T > set)
void insert(Stack &stack, Node *node)
Definition: avl_GenTree.cpp:256
DLNode * first(void) const
Definition: DLList.h:93
Definition: concepts.h:1079
void enable() override
Definition: alloc_ListGC.cpp:136
const T & first(void) const
Definition: MessageException.h:30
void insert(const T &item)
void add(const T &value)
Definition: List.h:131
Set< T > operator&(const Set &set)
Definition: SimpleGC.h:34
void removeBefore(const Iter< T > &pos)
void copy(const Collection< T > &items)
void print(io::Output &out)
Definition: checksum_MD5.cpp:328
const T & last(void) const
void exchange(Node *n, Node *m)
Definition: avl_GenTree.cpp:195
IntFormat width(int width, IntFormat fmt)
Definition: Output.h:261
typename type_info< T >::out_t out
Definition: type_info.h:284
void * allocate(t::size size)
Definition: alloc_DefaultAllocator.cpp:131
void removeByKey(const K &key)
void addBefore(const Iter< T > &pos, const T &item)
Definition: concepts.h:348
bool atEnd(void) const
Definition: DLList.h:37
char buffer[0]
Definition: StackAllocator.h:58
bool equals(const MutableIter &iterator) const
@ RIGHT
Definition: Output.h:45
const char * chars(void) const
Definition: String.h:76
void insert(int index, const T &item)
Definition: util_WAHVector.cpp:157
static void dump(Tree::Node *node, int tab=0)
Definition: avl_Tree.cpp:64
~ListGC()
Definition: alloc_ListGC.cpp:76
t::uint32 sum(void)
Definition: checksum_Fletcher.cpp:100
Printable< T, M > p(const T &data, const M &man)
Definition: Output.h:302
bool operator<=(const Set &coll)
virtual int flush(void)
Definition: checksum_Fletcher.cpp:150
void addFirst(DLNode *node)
Definition: DLList.h:106
Definition: concepts.h:1323
Definition: AbstractGC.h:31
free_t * free_list
Definition: BlockAllocatorWithGC.h:62
int count(void) const
Definition: Vector.h:81
virtual bool mark(void *data, t::size size)
Definition: alloc_GroupedGC.cpp:352
virtual ~AbstractGC()
Definition: alloc_AbstractGC.cpp:90
static const int GREATER
Definition: concepts.h:976
Definition: concepts.h:443
Set< T > operator|=(const Set< T > set)
bool isEqual(const T &object1, const T &object2)
Definition: alloc_DefaultAllocator.cpp:77
void addAll(const Collection< T > &items)
@ LEFT
Definition: Output.h:43
Node * _right
Definition: GenTree.h:57
void free()
Definition: alloc_ListGC.cpp:46
Definition: AbstractGC.h:38
void meet(const Set< T > &set)
Set< T > operator|(const Set &set)
unsigned short uint16
Definition: arch.h:29
free_t * next
Definition: BlockAllocatorWithGC.h:61
void removeAll(const Collection< T > &items)
Set< T > operator-=(const Set< T > set)
bool isEmpty(void) const
Definition: StaticStack.h:41
virtual void clean(void *p)
Definition: alloc_AbstractGC.cpp:54
void put(const K &key, const T &value)
Definition: concepts.h:228
Node * leftMost(Stack &s, Node *n)
Definition: avl_GenTree.cpp:443
Iterable< KeyIter > keys() const
void doGC(void)
Definition: alloc_SimpleGC.cpp:121
static bool lessThan(const T &v1, const T &v2)
static bool equals(const T &val1, const T &val2)
Definition: concepts.h:1121
bool contains(const T &item)
MutableIter & operator=(const MutableIter &iterator)
const T & max(const T &x, const T &y)
Definition: compare.h:108
void * allocate()
Definition: StackAllocator.h:40
@ NONE
Definition: Output.h:42
void * allocate(void)
Definition: alloc_BlockAllocatorWithGC.cpp:69
void addLast(const T &item)
Iter(const Collection< T > &collection)
unsigned char digest_t[16]
Definition: MD5.h:36
static const int EQUAL
Definition: concepts.h:966
virtual int flush(void)
Definition: checksum_MD5.cpp:403
static bool equals(const T &v1, const T &v2)
Definition: alloc_ListGC.cpp:38
void free(void *block)
Definition: alloc_BlockAllocatorWithGC.cpp:117
MutableIter & operator++(int)
void mark()
Definition: alloc_ListGC.cpp:55
void clear(int i)
Definition: Flags.h:34
IntFormat bin(IntFormat fmt)
Definition: Output.h:257
SimpleGC(t::size size=4096)
Definition: alloc_SimpleGC.cpp:84
block_t * next
Definition: alloc_ListGC.cpp:58
const T & pop(void)
Definition: StaticStack.h:43
Definition: concepts.h:893
Definition: concepts.h:1362
Node * get(Node *node)
Definition: avl_Tree.cpp:123
Definition: concepts.h:1340
Node * _left
Definition: GenTree.h:57
void clear(void)
Definition: alloc_GroupedGC.cpp:171
bool operator==(const Collection &coll)
const T & operator[](int index) const
virtual void * allocate(t::size size)
Definition: alloc_GroupedGC.cpp:276
Definition: DefaultAllocator.h:32
bool equals(const Iter &iterator) const
T t
Definition: concepts.h:234
MutableCollection & operator=(const Collection< T > &c)
Iter< T > find(const T &item)
Definition: BlockInStream.h:31
virtual int write(const char *buffer, int size)
Definition: checksum_Fletcher.cpp:142
virtual void destroy(void *p)
Definition: alloc_BlockAllocatorWithGC.cpp:236
void addAfter(const Iter< T > &pos, const T &item)
KeyIter(const Map< K, T > &map)
Definition: concepts.h:1055
Set< T > operator+(const Set &set)
void map(const C &c, const F &f, D &d)
Definition: util.h:89
void runGC() override
Definition: alloc_ListGC.cpp:95
IntFormat pad(char pad, IntFormat fmt)
Definition: Output.h:266
def ones(v)
Definition: ones.py:3
const T & min(const T &x, const T &y)
Definition: compare.h:104
Definition: concepts.h:1403
Option< const T & > get(const K &key) const
Definition: concepts.h:152
static const int LESS
Definition: concepts.h:971
BackIterator & operator=(const BackIterator< T > &iter)
PairIterator(const Map< K, T > &map)
Node * root(void) const
Definition: BinTree.h:90
Definition: concepts.h:960
char * alloc(int size)
Definition: block_DynBlock.cpp:139
typename type_info< T >::in_t in
Definition: type_info.h:283
dir_t
Definition: GenTree.h:46
void quicksort(A &array, const C &c=Comparator< typename A::t >())
Definition: quicksort.h:30
void remove(const Collection< T >::Iter &iter)
static block_t * block(void *p)
Definition: alloc_ListGC.cpp:50
virtual void free(Node *node)
Definition: avl_Tree.cpp:363
MutableCollection< T > & operator+=(const T &item)
virtual void collect(AbstractGC &gc)=0
Definition: concepts.h:278
T & operator[](const K &k)
const rtti::Type & type_of(void)
Definition: type_of.h:77
Node * _right(void) const
Definition: Tree.h:39
AutoStringStartup & _
Definition: debug_CrashHandler.cpp:232
Definition: StackAllocator.h:56
Collection< T > self_t
Definition: concepts.h:239
virtual void endGC(void)
Definition: alloc_BlockAllocatorWithGC.cpp:227
int length(void) const
Definition: String.h:75
const T & operator[](const K &k) const
Fletcher(void)
Definition: checksum_Fletcher.cpp:45
bool bit(int i) const
Definition: BitVector.h:41
void put(const char *block, int size)
Definition: block_DynBlock.cpp:57
virtual cstring lastErrorMessage(void)
Definition: checksum_Fletcher.cpp:157
Definition: BlockAllocatorWithGC.h:61
virtual ~Fletcher(void)
Definition: checksum_Fletcher.cpp:164
Iterator(const BiDiList< T > &list)
def crange(c1, c2)
Definition: Char.py:20
int length(void) const
Definition: Vector.h:116
bool operator>=(const Set &coll)
Definition: concepts.h:931
Set< T > operator*(const Set &set)
int size(void) const
Definition: DynBlock.h:21
Node * _left(void) const
Definition: Tree.h:38
ListPrinter< T > list(const T &l, cstring s="", typename ListPrinter< T >::fun_t f=ListPrinter< T >::asis)
Definition: Output.h:321
void clean(void)
Definition: Tree.h:86
static const int UNCOMP
Definition: concepts.h:981
const T & get(const K &key, const T &def) const
Definition: Tree.h:57
Definition: concepts.h:1170
Iter & operator=(const Iter &iterator)
void put(const void *buffer, t::uint32 length)
Definition: checksum_MD5.cpp:125
name
Definition: Char.py:6
void put(io::InStream &in)
Definition: checksum_Fletcher.cpp:78
ListGC(GCManager &m, int limit=1024)
Definition: alloc_ListGC.cpp:66
Definition: concepts.h:574
int doCompare(const T &object1, const T &object2)
def set(self, s)
Definition: Char.py:10
virtual void free(void *block)
Definition: alloc_AbstractGC.cpp:125
static const K & key(const T &value)
int indexOf(const T &value, int start=0) const
const T & head(void) const
words
Definition: Char.py:7
void add(const T &v)
Definition: Vector.h:101
Set< T > operator*=(const Set< T > set)
GCManager & manager
Definition: AbstractGC.h:56
void clean() override
Definition: alloc_ListGC.cpp:143
void remove(void)
Definition: DLList.h:57
const T & top(void) const
Definition: Vector.h:170
void rotateRight(Stack &s)
Definition: avl_GenTree.cpp:213
static int compare(const T &object1, const T &object2)
static StackAllocator DEFAULT
Definition: StackAllocator.h:36
virtual cstring lastErrorMessage(void)
Definition: checksum_MD5.cpp:410
virtual ~GroupedGC(void)
Definition: alloc_GroupedGC.cpp:164
Definition: concepts.h:1389
Definition: concepts.h:1027
Definition: concepts.h:1230
uint64 size
Definition: arch.h:35
const EOL endl
Definition: io_Output.cpp:880
bool operator!=(const Collection &coll)
Definition: DefaultAllocator.h:39
t::uint32 computeHash(const T &object)
virtual ~MD5(void)
Definition: checksum_MD5.cpp:114
bool operator<(const Collection &coll)
virtual void collect(void)
Definition: GroupedGC.h:44
def __init__(self, name, s="")
Definition: Char.py:5
Definition: BitVector.h:133
Definition: concepts.h:625
const T & operator[](int i) const
balance_t _bal
Definition: GenTree.h:58
Definition: util_WAHVector.cpp:60
void disable() override
Definition: alloc_ListGC.cpp:131
void set(int index) const
Definition: BitVector.h:60
void * data()
Definition: alloc_ListGC.cpp:54
static int abs(int x)
Definition: avl_Tree.cpp:36
void unmark()
Definition: alloc_ListGC.cpp:56
void set(int index, const T &item)
static block_t * alloc(t::size s)
Definition: alloc_ListGC.cpp:42
GroupedGC(t::size size=4096)
Definition: alloc_GroupedGC.cpp:62
static DefaultAllocator DEFAULT
Definition: DefaultAllocator.h:41
virtual ~BadAlloc(void)
Definition: alloc_DefaultAllocator.cpp:104
Definition: concepts.h:729
elm::sys::Path Path
Definition: Path.h:188
virtual void endGC(void)
Definition: alloc_GroupedGC.cpp:413
void diff(const Set< T > &set)
virtual void endGC(void)
Definition: alloc_SimpleGC.cpp:231
unsigned char uint8
Definition: arch.h:27
virtual ~AbstractBlockAllocatorWithGC(void)
Definition: alloc_BlockAllocatorWithGC.cpp:48
virtual ~GCManager()
Definition: alloc_AbstractGC.cpp:37
virtual void collect(void)=0
void get(char *block, int size, int pos)
Definition: block_DynBlock.cpp:77
void clear(void)
Definition: alloc_StackAllocator.cpp:109
AbstractBlockAllocatorWithGC(t::size block_size, t::size chunk_size=1<< 20)
Definition: alloc_BlockAllocatorWithGC.cpp:37
BackIterator(const BiDiList< T > &list)
Iterator & operator=(const BackIterator< T > &iter)
int free_cnt
Definition: BlockAllocatorWithGC.h:63
Definition: SimpleGC.h:58
Definition: concepts.h:784
static t::uint32 hash(const T &object)
void join(const Set< T > &set)
unsigned int uint32
Definition: arch.h:31
Definition: SegmentBuilder.h:30
Definition: concepts.h:75
static int compare(const T &v1, const T &v2)
virtual void beginGC(void)
Definition: alloc_GroupedGC.cpp:385
static bool equals(const T &object1, const T &object2)
char * mark_t
Definition: StackAllocator.h:45
mark_t mark(void)
Definition: alloc_StackAllocator.cpp:142
virtual int compare(Node *node1, Node *node2)=0
Definition: concepts.h:1329
void removeAfter(const Iter< T > &pos)
void remove(const T &item)
virtual ~Temp(void)
Definition: alloc_SimpleGC.cpp:46
static bool isEqual(const T &val1, const T &val2)
void remove(Stack &stack, Node *n)
Definition: avl_GenTree.cpp:318
Definition: IOException.h:29
dir_t topDir(void) const
Definition: GenTree.h:66
bool isMarked() const
Definition: alloc_ListGC.cpp:57
t::hash hash(const T &x)
Definition: hash.h:155
virtual bool mark(void *data, t::size size)
Definition: alloc_DefaultAllocator.cpp:148
bool operator!=(const MutableIter &iterator) const
void rotateLeft(Stack &s)
Definition: avl_GenTree.cpp:234
void digest(digest_t tab)
Definition: checksum_MD5.cpp:318
bool mark(void *data, t::size size)
Definition: alloc_SimpleGC.cpp:182
void newChunk(void)
Definition: alloc_StackAllocator.cpp:121
const T & top(void) const
bool containsAll(const C< T > &collection)
void * ptr
Definition: types.h:30
bool operator>(const Set &coll)
Set< T > operator&=(const Set< T > set)
virtual void collect(void)=0
virtual String message(void)
Definition: alloc_DefaultAllocator.cpp:109
int totalCount(void) const
Definition: alloc_BlockAllocatorWithGC.cpp:244
bool mark(void *data, t::size size) override
Definition: alloc_ListGC.cpp:123
void push(Node *node, dir_t dir)
Definition: GenTree.h:64
string str(const char *s)
Definition: String.h:150
virtual ~SimpleGC(void)
Definition: alloc_SimpleGC.cpp:105
Definition: BitVector.h:31
void clear(void)
Definition: alloc_SimpleGC.cpp:112
IntFormat right(IntFormat fmt)
Definition: Output.h:264
static const t::intptr TAG
Definition: alloc_ListGC.cpp:40
Definition: concepts.h:675
bool operator!=(const Iter &iterator) const
void set(int i)
Definition: Flags.h:33
IntFormat hex(IntFormat fmt)
Definition: Output.h:259
bool operator==(const Iter &iterator) const
IntFormat left(IntFormat fmt)
Definition: Output.h:263
bool equals(const Collection &coll)
T & operator[](int index)
Temp(SimpleGC &gc)
Definition: alloc_SimpleGC.cpp:40
void * allocate(t::size size)
Definition: alloc_SimpleGC.cpp:152
static bool greaterThan(const T &v1, const T &v2)
bool mark(void *ptr)
Definition: alloc_BlockAllocatorWithGC.cpp:191
bool operator==(const MutableIter &iterator) const
bool hasKey(const K &key) const
virtual void beginGC(void)
Definition: alloc_SimpleGC.cpp:205
K key_t
Definition: concepts.h:1368
bool subsetOf(const Set &coll)
virtual ~StackAllocator(void)
Definition: alloc_StackAllocator.cpp:63
void doGC(void)
Definition: alloc_GroupedGC.cpp:180
void release(mark_t mark)
Definition: alloc_StackAllocator.cpp:151
struct chunk_t * next
Definition: StackAllocator.h:57
void * allocate(t::size size) override
Definition: alloc_ListGC.cpp:81
const T & get(int index) const
Node * topNode(void) const
Definition: GenTree.h:67
MD5(void)
Definition: checksum_MD5.cpp:99
Node ** link(const Stack &s)
Definition: avl_GenTree.cpp:180
int lastIndexOf(const T &value, int start=-1) const
void set(const Iterator< T > &pos, const T &item)
void collectGarbage(void)
Definition: alloc_BlockAllocatorWithGC.cpp:124
uint64 intptr
Definition: arch.h:38
Iterable< PairIter > pairs() const
Set< T > operator-(const Set &set)
uint64 offset
Definition: arch.h:36
virtual void beginGC(void)
Definition: alloc_BlockAllocatorWithGC.cpp:218
void addFirst(const T &item)
StackAllocator(t::size size=4096)
Definition: alloc_StackAllocator.cpp:55
void setRoot(Node *node)
Definition: BinTree.h:93
virtual int write(const char *buffer, int size)
Definition: checksum_MD5.cpp:395
Definition: InStream.h:29
def dump(self)
Definition: Char.py:15