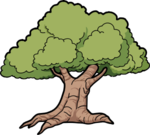 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_ALLOC_GROUPEDGC_H_
22 #define ELM_ALLOC_GROUPEDGC_H_
24 #include <elm/util/BitVector.h>
25 #include <elm/stree/Tree.h>
26 #include <elm/data/List.h>
27 #include <elm/data/BiDiList.h>
28 #include <elm/alloc/DefaultAllocator.h>
44 virtual void collect(
void) { ASSERTP(
false,
"collect function must be implemented."); }
45 virtual void endGC(
void);
49 void newChunk(
int index);
50 void *allocFromFreeList(
t::size size,
unsigned int index);
52 typedef struct block_t {
58 typedef struct chunk_t {
67 List<chunk_t *> chunks;
70 inhstruct::DLList temps;
73 typedef stree::Tree<void *, chunk_t *> tree_t;
76 static inline t::size round(
t::size size) {
return (
size +
sizeof(block_t) - 1) & ~(
sizeof(block_t) - 1); }
79 unsigned int shiftToIndex;
84 unsigned long totalChunkSize;
86 unsigned int currentMaxIndex;
87 unsigned int maxSlotSize;
90 unsigned long* useDist;
91 unsigned long* currUseDist;
92 unsigned long* markDist;
93 unsigned long* currMarkDist;
94 unsigned long* freeDist;
95 unsigned long* currFreeDist;
96 unsigned long* chunkDist;
97 unsigned long* gcDist;
100 unsigned int maxAllocatableIndex;
101 chunk_t **chunk_list;
103 unsigned long* blockCount;
105 unsigned int requestCount;
106 unsigned int requestThreshold;
109 unsigned int allocFuncCount;
110 unsigned int allocCount;
111 unsigned int markCount;
112 unsigned int gcTimes;
113 unsigned long totalAllocTime;
virtual bool mark(void *data, t::size size)
Definition: alloc_GroupedGC.cpp:352
friend class TempGroupedGC
Definition: GroupedGC.h:33
void clear(void)
Definition: alloc_GroupedGC.cpp:171
virtual void * allocate(t::size size)
Definition: alloc_GroupedGC.cpp:276
Definition: GroupedGC.h:32
bool getNeedGC(void)
Definition: GroupedGC.h:46
virtual ~GroupedGC(void)
Definition: alloc_GroupedGC.cpp:164
uint64 size
Definition: arch.h:35
Definition: DefaultAllocator.h:39
virtual void collect(void)
Definition: GroupedGC.h:44
GroupedGC(t::size size=4096)
Definition: alloc_GroupedGC.cpp:62
virtual void endGC(void)
Definition: alloc_GroupedGC.cpp:413
unsigned char uint8
Definition: arch.h:27
virtual void beginGC(void)
Definition: alloc_GroupedGC.cpp:385
void setDisableGC(bool b)
Definition: GroupedGC.h:41
void doGC(void)
Definition: alloc_GroupedGC.cpp:180
uint64 intptr
Definition: arch.h:38