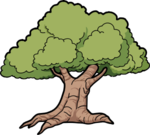 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
28 #include <elm/type_info.h>
29 #include <elm/util/misc.h>
36 template <
class T>
class fast {
38 static inline void copy(T *target,
const T *source,
int size)
39 { ::memcpy(target, source,
size *
sizeof(T)); }
40 static inline void move(T *target,
const T *source,
int size)
41 { ::memmove(target, source,
size *
sizeof(T)); }
43 { ::memset(target, 0,
size *
sizeof(T)); }
44 static inline bool equals(
const T* t1,
const T* t2,
int size)
45 { return ::memcmp(t1, t2,
size) == 0; }
51 template <
class T>
class slow {
53 static inline void copy(T *target,
const T *source,
int size)
54 {
for(
int i = 0; i <
size; i++) target[i] = source[i]; }
56 {
for(
int i =
size - 1; i >= 0; i--) target[i] = source[i]; }
57 static inline void move(T *target,
const T *source,
int size)
60 {
for(
int i = 0; i <
size; i++) target[i] = T(); }
61 static inline bool equals(
const T* t1,
const T* t2,
int size)
62 {
for(
int i = 0; i <
size; i++)
if(!(t1[i] == t2[i]))
return false;
return true; }
64 {
for(
int i = 0; i <
size; i++) ::
new((
void *)(t + i)) T(); }
66 {
for(
int i = 0; i <
size; i++) (t + i)->~T(); }
70 template <
class T>
inline void copy(T *target,
const T *source,
int size)
72 template <
class T>
inline void copy_back(T *target,
const T *source,
int size)
73 {
for(
int i =
size - 1; i >= 0; i--) target[i] = source[i]; }
74 template <
class T>
inline void move(T *target,
const T *source,
int size)
76 template <
class T>
inline void set(T *target,
int size,
const T& v)
77 {
for(
int i = 0; i <
size; i++) target[i] = v; }
78 template <
class T>
inline void clear(T *target,
int size)
80 template <
class T>
inline bool equals(
const T* t1,
const T* t2,
int size)
87 {
copy(
reinterpret_cast<char *
>(d),
reinterpret_cast<char *
>(a),
sizeof(
cstring) * s); }
89 {
move(
reinterpret_cast<char *
>(d),
reinterpret_cast<char *
>(a),
sizeof(
cstring) * s); }
91 {
clear(
reinterpret_cast<char *
>(d),
sizeof(
cstring) * s); }
95 template <
class T>
void reverse(T *a,
int n)
96 {
for(
int i = 0; i < n / 2; i++)
swap(a[i], a[n - 1 - i]); }
void copy(T *target, const T *source, int size)
Definition: array.h:70
void copy_back(T *target, const T *source, int size)
Definition: array.h:72
static bool equals(const T *t1, const T *t2, int size)
Definition: array.h:61
static bool equals(const T *t1, const T *t2, int size)
Definition: array.h:44
static void destruct(T *t, int size)
Definition: array.h:47
static void copy(T *target, const T *source, int size)
Definition: array.h:53
static void destruct(T *t, int size)
Definition: array.h:65
bool equals(const T *t1, const T *t2, int size)
Definition: array.h:80
static void construct(T *t, int size)
Definition: array.h:46
void clear(T *target, int size)
Definition: array.h:78
void set(T *target, int size, const T &v)
Definition: array.h:76
uint64 size
Definition: arch.h:35
static void construct(T *t, int size)
Definition: array.h:63
static void clear(T *target, int size)
Definition: array.h:59
void move(T *target, const T *source, int size)
Definition: array.h:74
void construct(T *t, int size)
Definition: array.h:82
static void copy(T *target, const T *source, int size)
Definition: array.h:38
static void copy_back(T *target, const T *source, int size)
Definition: array.h:55
void swap(T &x, T &y)
Definition: misc.h:27
void destruct(T *t, int size)
Definition: array.h:84
static void move(T *target, const T *source, int size)
Definition: array.h:57
static void clear(T *target, int size)
Definition: array.h:42
static void move(T *target, const T *source, int size)
Definition: array.h:40
void reverse(T *a, int n)
Definition: array.h:95