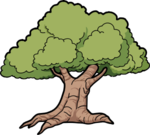 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
25 #include <elm/types.h>
26 #include <elm/string.h>
27 #include <elm/util/Option.h>
28 #include <elm/util/Pair.h>
29 #include <elm/equiv.h>
33 namespace sys {
class Path; }
55 inline bool isEqual(
const T& key1,
const T& key2)
const {
return equals(key1, key2); }
62 static inline bool equals(
int key1,
int key2) {
return key1 == key2; }
64 inline bool isEqual(
int key1,
int key2)
const {
return equals(key1, key2); }
71 static inline bool equals(T *key1, T *key2) {
return key1 == key2; }
73 inline bool isEqual(T *key1, T *key2)
const {
return equals(key1, key2); }
80 static inline bool equals(
const T *key1,
const void *key2)
81 {
return key1 == key2; }
83 inline bool isEqual(
const T *key1,
const T *key2)
const {
return equals(key1, key2); }
99 inline bool isEqual(
string key1,
string key2)
const {
return equals(key1, key2); }
106 static inline bool equals(
const T& p1,
const T& p2) {
return p1 == p2; };
108 inline bool isEqual(
const T& key1,
const T& key2)
const {
return equals(key1, key2); }
120 inline operator t::hash(
void)
const {
return h; }
130 static bool equals(
const T& v1,
const T& v2) {
return v1 == v2; }
132 inline bool isEqual(
const T& key1,
const T& key2)
const {
return equals(key1, key2); }
136 template <
class K,
class T,
class H = HashKey<K> >
144 inline bool isEqual(
const t& k1,
const t& k2)
const {
return H::isEqual(k1.fst, k2.fst); }
t::hash computeHash(T *key) const
Definition: hash.h:72
static bool equals(const String &key1, const String &key2)
Definition: hash.h:97
static bool equals(const T &p1, const T &p2)
Definition: hash.h:106
bool isEqual(cstring key1, cstring key2) const
Definition: hash.h:91
const char * chars(void) const
Definition: String.h:76
Printable< T, M > p(const T &data, const M &man)
Definition: Output.h:302
static bool equals(int key1, int key2)
Definition: hash.h:62
t::hash computeHash(const sys::Path &key) const
Definition: hash.h:151
t::hash hash(void) const
Definition: hash.h:119
H & keyHash()
Definition: hash.h:141
const H & keyHash() const
Definition: hash.h:140
bool equals(const C1 &c1, const C2 &c2)
Definition: util.h:107
Hasher & operator<<(const T &value)
Definition: hash.h:118
static bool equals(const T &key1, const T &key2)
Definition: hash.h:53
static bool equals(CString key1, CString key2)
Definition: hash.h:89
t::hash computeHash(const T &key) const
Definition: hash.h:131
bool isEqual(const T *key1, const T *key2) const
Definition: hash.h:83
t::hash hash_ptr(const void *p)
Definition: hash.h:40
void add(const T &value)
Definition: hash.h:116
bool isEqual(int key1, int key2) const
Definition: hash.h:64
static t::hash hash(const T &v)
Definition: hash.h:129
bool isEqual(T *key1, T *key2) const
Definition: hash.h:73
static t::hash hash(CString key)
Definition: hash.h:88
Hasher(void)
Definition: hash.h:115
int length(void) const
Definition: String.h:75
static t::hash hash(T *key)
Definition: hash.h:70
bool isEqual(const T &key1, const T &key2) const
Definition: hash.h:108
static bool equals(const T *key1, const void *key2)
Definition: hash.h:80
const char * chars(void) const
Definition: CString.h:27
bool isEqual(const T &key1, const T &key2) const
Definition: hash.h:132
Pair< T1, T2 > T
Definition: hash.h:104
t::hash computeHash(const T *key) const
Definition: hash.h:82
static t::hash hash(const String &key)
Definition: hash.h:96
bool isEqual(const T &key1, const T &key2) const
Definition: hash.h:55
t::hash computeHash(const T &key) const
Definition: hash.h:107
bool isEqual(const t &k1, const t &k2) const
Definition: hash.h:144
uint64 size
Definition: arch.h:35
t::hash hash_jenkins(const void *block, int size)
Definition: util_HashKey.cpp:92
static bool equals(const T &v1, const T &v2)
Definition: hash.h:130
Pair< K, T > t
Definition: hash.h:139
t::hash computeHash(cstring key) const
Definition: hash.h:90
static bool equals(T *key1, T *key2)
Definition: hash.h:71
t::hash computeHash(int key) const
Definition: hash.h:63
bool isEqual(const sys::Path &key1, const sys::Path &key2) const
Definition: hash.h:152
Hasher & operator+=(const T &value)
Definition: hash.h:117
t::hash hash(const T &x)
Definition: hash.h:155
static t::hash hash(const T *key)
Definition: hash.h:79
static t::hash hash(const T &p)
Definition: hash.h:105
t::intptr hash
Definition: hash.h:34
t::hash computeHash(string key) const
Definition: hash.h:98
t::hash computeHash(const T &key) const
Definition: hash.h:54
bool hash_equals(const void *p1, const void *p2, int size)
Definition: util_HashKey.cpp:160
static t::hash hash(int key)
Definition: hash.h:61
bool isEqual(string key1, string key2) const
Definition: hash.h:99
t::hash hash_string(const char *chars, int length)
Definition: util_HashKey.cpp:121
static t::hash hash(const T &key)
Definition: hash.h:52
t::hash hash_cstring(const char *chars)
Definition: util_HashKey.cpp:139
uint64 intptr
Definition: arch.h:38
static bool equals(const T &v1, const T &v2)
Definition: equiv.h:35
elm::t::hash computeHash(const t &k) const
Definition: hash.h:143