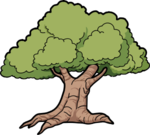 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_DATA_TREEBAG_H
22 #define ELM_DATA_TREEBAG_H
24 #include <elm/utility.h>
25 #include <elm/PreIterator.h>
26 #include <elm/data/Vector.h>
27 #include <elm/data/VectorQueue.h>
28 #include <elm/inhstruct/BinTree.h>
29 #include <elm/compare.h>
30 #include <elm/adapter.h>
34 template <
class T,
class C = Comparator<T>,
class A = DefaultAlloc >
43 {
return t->A::allocate(
size); }
45 { this->~Node();
t->A::free(
this); }
59 inline int count(
void)
const {
return root.count(); }
60 inline bool contains(
const T& x)
const {
return find(x) !=
nullptr; }
65 {
for(
const auto x: c)
if(!
contains(x))
return false;
return true; }
72 {
if(tree.root.
root()) downLeft(
_(tree.root.
root())); }
73 bool ended(
void)
const {
return !s; }
75 {
if(s.
top()->right()) downLeft(
_(s.
top()->right()));
76 else { Node *n = s.
pop();
if(s && n == s.
top()->right()) upRight(n); } }
77 const T&
item(
void)
const {
return s.
top()->val; }
78 inline bool equals(
const Iter& i)
const {
return s == i.s; }
82 inline void downLeft(Node *n)
84 inline void upRight(Node *n)
85 {
while(s && s.
top()->right() == n) n = s.
pop(); }
89 inline Iter
begin()
const {
return Iter(*
this); }
90 inline Iter
end()
const {
return Iter(); }
111 Node *node = todo.
get();
113 todo.
put((Node *)node->left());
115 todo.
put((Node *)node->right());
122 Node *node = (Node *)root.
root();
123 Node *new_node =
new(
this) Node(x);
128 int cmp = C::doCompare(x, node->val);
131 node->insertRight(new_node);
135 node = (Node *)node->right();
139 node->insertLeft(new_node);
143 node = (Node *)node->left();
148 template <
class CC>
void addAll (
const CC &c)
149 {
for(
const auto x: c)
add(x); }
151 Node *node = (Node *)root.
root(), *parent = 0;
154 int cmp = C::compare(x, node->val);
159 node = (Node *)node->right();
161 node = (Node *)node->left();
163 Node *
left = (Node *)node->left(), *
right = (Node *)node->right();
166 replace(parent, node,
right);
168 replace(parent, node,
left);
170 replace(parent, node,
left);
171 for(node =
left; node->right(); node = (Node *)node->right());
172 node->insertRight(
right);
177 {
for(
const auto x: c)
remove(x); }
186 const T *
find(
const T& x)
const {
187 Node *node = (Node *)root.
root();
189 int cmp = C::compare(x, node->val);
193 node = (Node *)node->right();
195 node = (Node *)node->left();
203 void replace(Node *parent, Node *old, Node *_new) {
206 else if(parent->left() == old)
207 parent->insertLeft(_new);
209 parent->insertRight(_new);
215 #endif // ELM_GENSTRUCT_SORTEDBINTREE_H
const C & comparator() const
Definition: TreeBag.h:54
Definition: util_WAHVector.cpp:157
void next(void)
Definition: TreeBag.h:74
Iter(const TreeBag &tree)
Definition: TreeBag.h:71
const T & item(void) const
Definition: TreeBag.h:77
const T & get(void)
Definition: VectorQueue.h:114
void add(const T &x)
Definition: TreeBag.h:121
C & comparator()
Definition: TreeBag.h:55
void put(const T &value)
Definition: VectorQueue.h:104
void remove(const T &x)
Definition: TreeBag.h:150
Iter begin() const
Definition: TreeBag.h:89
Iter end() const
Definition: TreeBag.h:90
void push(const T &v)
Definition: Vector.h:173
Definition: VectorQueue.h:31
bool containsAll(const CC &c) const
Definition: TreeBag.h:64
bool equals(const TreeBag< T, C > &t) const
Definition: TreeBag.h:92
TreeBag(const TreeBag< T, C > &t)
Definition: TreeBag.h:52
void addAll(const CC &c)
Definition: TreeBag.h:148
Node * root(void) const
Definition: BinTree.h:90
void copy(const TreeBag< T, C > &t)
Definition: TreeBag.h:180
bool operator!=(const TreeBag< T, C > &t) const
Definition: TreeBag.h:102
T t
Definition: compare.h:36
bool ended(void) const
Definition: TreeBag.h:73
AutoStringStartup & _
Definition: debug_CrashHandler.cpp:232
void free(t::ptr p) const
Definition: custom.h:32
int count(void) const
Definition: TreeBag.h:59
void removeAll(const CC &c)
Definition: TreeBag.h:176
bool isEmpty(void) const
Definition: TreeBag.h:61
const T & top(void) const
Definition: Vector.h:170
void remove(const Iter &iter)
Definition: TreeBag.h:178
bool contains(const T &x) const
Definition: TreeBag.h:60
bool equals(const Iter &i) const
Definition: TreeBag.h:78
A & allocator()
Definition: TreeBag.h:56
uint64 size
Definition: arch.h:35
bool isEmpty(void) const
Definition: BinTree.h:81
~TreeBag(void)
Definition: TreeBag.h:53
TreeBag()
Definition: TreeBag.h:51
IntFormat right(IntFormat fmt)
Definition: Output.h:264
const T * find(const T &x) const
Definition: TreeBag.h:186
IntFormat left(IntFormat fmt)
Definition: Output.h:263
bool operator==(const TreeBag< T, C > &t) const
Definition: TreeBag.h:101
void clear(void)
Definition: TreeBag.h:105
T pop(void)
Definition: Vector.h:172
void setRoot(Node *node)
Definition: BinTree.h:93