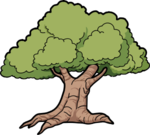 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_AVL_GENTREE_H
22 #define ELM_AVL_GENTREE_H
24 #include <elm/utility.h>
25 #include <elm/PreIterator.h>
26 #include <elm/adapter.h>
27 #include <elm/array.h>
28 #include <elm/data/StaticStack.h>
29 #include <elm/data/Vector.h>
35 namespace elm {
namespace avl {
70 Node **
link(
const Stack& s);
72 void insert(Stack& stack, Node *node);
75 void remove(Stack& stack, Node *n);
81 # ifdef ELM_AVL_INVARIANT
84 virtual int cmp(Node *n1, Node *n2)
const = 0;
86 bool invariant(Node *n,
int& h, Node *&
max, Node *&
min);
87 bool invariant(Node *n);
88 Node *pointed(
const Stack& s)
const;
96 template <
class T,
class K = IdAdapter<T>,
class C = elm::Comparator<
typename K::key_t>,
class A = DefaultAlloc>
106 inline const typename K::key_t&
key(
void)
const {
return K::key(
data); }
108 inline static void *
operator new(
size_t s, A *a) {
return a->allocate(s); }
109 inline void free(A& a) { this->~
Node(); a.free(
this); }
141 inline T *
get(
const typename K::key_t& key)
142 {
Node *node =
find(key);
if(!node)
return 0;
else return &node->data; }
143 inline const T *
get(
const typename K::key_t& key)
const
144 {
const Node *node =
find(key);
if(!node)
return 0;
else return &node->data; }
158 inline bool contains(
const typename K::key_t& item)
const {
return find(item) !=
nullptr; }
161 {
for(
const auto x: c)
if(!
contains(x))
return false;
return true; }
169 {
if(tree.
root()) visit(tree.
root()); }
175 if(last == s.
top()->left()) { s.
push(s.
top());
break; }
176 else if(last == s.
top() && s.
top()->right()) { visit(s.
top()->right());
break; }
179 inline const T&
item(
void)
const {
return s.
top()->data; }
182 inline T&
data(
void) {
return s.
top()->data; }
184 void visit(
Node *node) {
187 while(s.
top()->left()) s.
push(s.
top()->left());
192 inline Iter
begin(
void)
const {
return Iter(*
this); }
193 inline Iter
end(
void)
const {
return Iter(); }
197 for(; ai() && bi(); ai++, bi++)
198 if(!(C::doCompare(K::key(*ai), K::key(*bi)) == 0))
208 if(
root() !=
nullptr)
210 while(!s.isEmpty()) {
212 if(n->left() !=
nullptr)
214 if(n->right() !=
nullptr)
229 template <
class CC>
inline void addAll(
const CC& c)
230 {
for(
const auto x: c)
add(x); }
235 {
for(
const auto x: c)
remove(x); }
244 if(tree.
_root ==
nullptr)
248 VisitStack ss(tree.
root()), st(
root());
249 while(!ss.isEmpty()) {
250 if(ss.top()->left() !=
nullptr)
251 ss.copyLeft(
this, st);
253 while(ss.top()->right() ==
nullptr) {
261 while(ss.top()->right() == prv);
263 ss.copyRight(
this, st);
269 # ifdef ELM_AVL_INVARIANT
271 return compare(
static_cast<Node *
>(n1)->key(),
static_cast<Node *
>(n2)->key());
273 void print(
io::Output&
out, AbstractTree::Node *n)
const override {
274 out << static_cast<Node *>(n)->key();
283 if(n->left() ==
nullptr)
285 else if(n->right() ==
nullptr)
299 inline int compare(
const typename K::key_t& k1,
const typename K::key_t& k2)
const
300 {
return C::compare(k1, k2); }
304 while(
p !=
nullptr) {
305 int cmp =
compare(key, K::key(
p->data));
343 #endif // ELM_AVL_GENTREE_H
Node * root(void) const
Definition: GenTree.h:112
void insert(Stack &stack, Node *node)
Definition: avl_GenTree.cpp:256
VisitStack(void)
Definition: GenTree.h:121
bool operator!=(const GenTree< T, K, C > &tree) const
Definition: GenTree.h:203
void copyLeft(A *a, VisitStack &s)
Definition: GenTree.h:123
self_t & operator+=(const T &x)
Definition: GenTree.h:239
void exchange(Node *n, Node *m)
Definition: avl_GenTree.cpp:195
typename type_info< T >::out_t out
Definition: type_info.h:284
void remove(const T &x)
Definition: GenTree.h:232
Definition: util_WAHVector.cpp:157
Printable< T, M > p(const T &data, const M &man)
Definition: Output.h:302
const T * get(const typename K::key_t &key) const
Definition: GenTree.h:143
self_t & operator-=(const T &x)
Definition: GenTree.h:240
bool contains(const typename K::key_t &item) const
Definition: GenTree.h:158
A & allocator()
Definition: GenTree.h:139
Node * right(void)
Definition: GenTree.h:105
void remove(Stack &s, Node *n)
Definition: GenTree.h:280
int count(void) const
Definition: GenTree.h:41
T data
Definition: GenTree.h:107
Node * _right
Definition: GenTree.h:57
@ RIGHT
Definition: GenTree.h:49
bool isEmpty(void) const
Definition: StaticStack.h:41
Pair< T1, T2 > pair(const T1 &v1, const T2 &v2)
Definition: Pair.h:63
Node * leftMost(Stack &s, Node *n)
Definition: avl_GenTree.cpp:443
T2 snd
Definition: Pair.h:36
Node * _root
Definition: GenTree.h:78
static const int MAX_HEIGHT
Definition: GenTree.h:44
const T & max(const T &x, const T &y)
Definition: compare.h:108
Node(const T &item)
Definition: GenTree.h:102
void next(void)
Definition: GenTree.h:171
const C & comparator() const
Definition: GenTree.h:136
bool equals(const Iter &i) const
Definition: GenTree.h:180
const T & pop(void)
Definition: StaticStack.h:43
Node * _left
Definition: GenTree.h:57
void removeAll(const CC &c)
Definition: GenTree.h:234
Definition: GenTree.h:165
Iter begin(void) const
Definition: GenTree.h:192
bool isEmpty(void) const
Definition: GenTree.h:162
signed char balance_t
Definition: GenTree.h:45
Node * find(const typename K::key_t &key) const
Definition: GenTree.h:320
int count(void) const
Definition: GenTree.h:157
VisitStack(Node *n)
Definition: GenTree.h:122
const T & min(const T &x, const T &y)
Definition: compare.h:104
Definition: GenTree.h:114
int _cnt
Definition: GenTree.h:79
Definition: GenTree.h:119
void clear(void)
Definition: GenTree.h:206
AbstractTree(void)
Definition: GenTree.h:52
~GenTree(void)
Definition: GenTree.h:135
const T & item(void) const
Definition: GenTree.h:179
dir_t
Definition: GenTree.h:46
Definition: GenTree.h:100
Node(const Node *node)
Definition: GenTree.h:103
T t
Definition: compare.h:36
Node * topNode(void) const
Definition: GenTree.h:116
const K::key_t & key(void) const
Definition: GenTree.h:106
Stack(void)
Definition: GenTree.h:63
T * get(const typename K::key_t &key)
Definition: GenTree.h:141
T t
Definition: GenTree.h:130
Iter end(void) const
Definition: GenTree.h:193
bool containsAll(const CC &c) const
Definition: GenTree.h:160
void rotateRight(Stack &s)
Definition: avl_GenTree.cpp:213
void addAll(const CC &c)
Definition: GenTree.h:229
T & data(void)
Definition: GenTree.h:182
Iter()
Definition: GenTree.h:167
void add(const T &item)
Definition: GenTree.h:222
GenTree(void)
Definition: GenTree.h:133
GenTree< T, K, C, A > self_t
Definition: GenTree.h:131
balance_t _bal
Definition: GenTree.h:58
Definition: StaticStack.h:31
C & comparator()
Definition: GenTree.h:137
const A & allocator() const
Definition: GenTree.h:138
void copyRight(A *a, VisitStack &s)
Definition: GenTree.h:125
Iter(const self_t &tree)
Definition: GenTree.h:168
bool equals(const GenTree< T, K, C > &tree) const
Definition: GenTree.h:195
@ NONE
Definition: GenTree.h:48
unsigned int uint32
Definition: arch.h:31
int compare(const typename K::key_t &k1, const typename K::key_t &k2) const
Definition: GenTree.h:299
void free(A &a)
Definition: GenTree.h:109
void remove(Stack &stack, Node *n)
Definition: avl_GenTree.cpp:318
dir_t topDir(void) const
Definition: GenTree.h:66
void exchange(Node *n, Node *p)
Definition: GenTree.h:333
bool ended(void) const
Definition: GenTree.h:170
void rotateLeft(Stack &s)
Definition: avl_GenTree.cpp:234
void copy(const GenTree< T, K, C > &tree)
Definition: GenTree.h:242
void set(const T &item)
Definition: GenTree.h:145
Node(void)
Definition: GenTree.h:56
void push(Node *node, dir_t dir)
Definition: GenTree.h:64
@ LEFT
Definition: GenTree.h:47
void remove(const Iter &iter)
Definition: GenTree.h:237
IntFormat right(IntFormat fmt)
Definition: Output.h:264
IntFormat left(IntFormat fmt)
Definition: Output.h:263
void removeByKey(const typename K::key_t &item)
Definition: GenTree.h:148
Node * topNode(void) const
Definition: GenTree.h:67
Node ** link(const Stack &s)
Definition: avl_GenTree.cpp:180
bool operator==(const GenTree< T, K, C > &tree) const
Definition: GenTree.h:202
GenTree< T, K, C > & operator=(const GenTree< T, K, C > &tree)
Definition: GenTree.h:267
friend class Invariant
Definition: GenTree.h:39
Node * lookup(Stack &s, const typename K::key_t &key) const
Definition: GenTree.h:302
void push(const Node * &v)
Definition: StaticStack.h:44
const Pair< Node *, dir_t > & top(void) const
Definition: StaticStack.h:42
bool equals(const StaticStack< T, N > &s) const
Definition: StaticStack.h:71
Node * left(void)
Definition: GenTree.h:104
GenTree(const GenTree< T > &tree)
Definition: GenTree.h:134
T1 fst
Definition: Pair.h:35