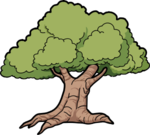 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_UTIL_PAIR_H
22 #define ELM_UTIL_PAIR_H
32 template <
class T1,
class T2>
38 inline Pair(
const T1& _fst,
const T2& _snd):
fst(_fst),
snd(_snd) { }
51 template <
class T1,
class T2>
54 inline RefPair(T1& r1, T2& r2): v1(r1), v2(r2) { }
typename type_info< T >::out_t out
Definition: type_info.h:284
Printable< T, M > p(const T &data, const M &man)
Definition: Output.h:302
RefPair< T1, T2 > & operator=(const Pair< T1, T2 > &p)
Definition: Pair.h:55
AutoString & operator<<(CString str, const T &value)
Definition: AutoString.h:75
Pair< T1, T2 > pair(const T1 &v1, const T2 &v2)
Definition: Pair.h:63
T2 snd
Definition: Pair.h:36
bool operator>(const Pair< T1, T1 > &pair) const
Definition: Pair.h:45
Pair(const T1 &_fst, const T2 &_snd)
Definition: Pair.h:38
typename type_info< T >::in_t in
Definition: type_info.h:283
RefPair< T1, T2 > let(T1 &v1, T2 &v2)
Definition: Pair.h:65
RefPair(T1 &r1, T2 &r2)
Definition: Pair.h:54
Pair(void)
Definition: Pair.h:37
bool operator!=(const Pair< T1, T2 > &pair) const
Definition: Pair.h:42
Pair(const Pair< T1, T2 > &pair)
Definition: Pair.h:39
bool operator==(const Pair< T1, T2 > &pair) const
Definition: Pair.h:41
bool operator<(const Pair< T1, T1 > &pair) const
Definition: Pair.h:43
Pair< T1, T2 > & operator=(const Pair< T1, T2 > &pair)
Definition: Pair.h:40
bool operator<=(const Pair< T1, T1 > &pair) const
Definition: Pair.h:44
io::StringInput operator>>(const string &str, T &val)
Definition: AutoString.h:82
bool operator>=(const Pair< T1, T1 > &pair) const
Definition: Pair.h:46
T1 fst
Definition: Pair.h:35