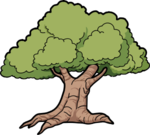 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_DATA_HASHTABLE_H_
22 #define ELM_DATA_HASHTABLE_H_
25 #include <elm/adapter.h>
26 #include <elm/array.h>
31 template <
class T,
class H = HashKey<T>,
class A = DefaultAlloc >
39 inline node_t(
const T& d): next(0), data(d) { }
45 node_t *
find(
const T& key)
const {
46 int i = H::computeHash(key) % _size;
47 for(node_t *node = _tab[i], *prev = 0; node; prev = node, node = node->next)
48 if(H::isEqual(node->data, key)) {
49 if(prev) { prev->next = node->next; node->next = _tab[i]; _tab[i] = node; }
56 int i = H::computeHash(key) % _size;
57 for(node_t *node = _tab[i], *prev = 0; node; prev = node, node = node->next)
58 if(H::isEqual(node->data, key)) {
66 node_t *make(
const T& data) {
67 int i = H::computeHash(data) % _size;
68 node_t *node =
new(A::allocate(
sizeof(node_t))) node_t(data);
74 struct InternIterator {
76 inline InternIterator(
const self_t& _htab): node(nullptr), htab(&_htab) { i = 0; step(); }
77 inline InternIterator(
const self_t& _htab,
bool end): node(nullptr), htab(&_htab)
78 {
if(
end) { i = htab->_size; node =
nullptr; }
else { i = 0; step(); } }
79 inline bool ended(
void)
const {
return i >= htab->_size; }
80 inline void next(
void) { node = node->next;
if(!node) { i++; step(); } }
81 inline bool equals(
const InternIterator& it)
const {
return node == it.node && i == it.i && htab == it.htab; }
85 inline void step(
void) {
for(; i < htab->_size; i++)
if(htab->_tab[i]) { node = htab->_tab[i];
break; } }
92 HashTable(
int _size = 211): _size(_size), _tab(new(A::
allocate(_size * sizeof(node_t *))) node_t *[_size])
97 {
clear();
delete [] _tab; }
98 inline const H&
hash()
const {
return *
this; }
99 inline H&
hash() {
return *
this; }
103 inline const T *
get(
const T& key)
const
104 { node_t *node =
find(key);
return node ? &node->data : 0; }
106 { node_t *node =
find_const(key);
return node ? &node->data : 0; }
108 { node_t *node =
find(key);
return node != 0; }
110 { node_t *node =
find_const(key);
return node != 0; }
115 { node_t *node =
find(data);
if(node) node->data = data;
else add(data); }
116 template <
class CC>
void putAll(
const CC& c)
117 {
for(
const auto x: c)
put(x); }
122 {
for(
int i = 0; i < _size; i++)
if(_tab[i])
return false;
return true; }
125 {
int cnt = 0;
for(
int i = 0; i < _size; i++)
for(node_t *cur = _tab[i]; cur; cur = cur->next) cnt++;
return cnt; }
127 {
return find(x) !=
nullptr; }
131 {
for(
const auto x: c)
if(!
contains(x))
return false;
return true; }
133 {
for(
const auto x: c)
if(!
contains_const(x))
return false;
return true; }
139 inline const T&
item(
void)
const {
return this->node->data; }
141 inline Iter
begin()
const {
return Iter(*
this); }
142 inline Iter
end()
const {
return Iter(*
this,
true); }
153 for(
int i = 0; i < _size; i++) {
154 for(node_t *cur = _tab[i], *next; cur; cur = next) { next = cur->next; A::free(cur); }
159 T *
add(
const T& data) {
return &make(data)->data; }
163 template <
class C>
void addAll(
const C& c)
164 {
for(
const auto x: c)
add(x); }
167 int i = H::computeHash(key) % _size;
168 for(node_t *node = _tab[i], *prev = 0; node; prev = node, node = node->next)
169 if(H::isEqual(node->data, key)) {
171 prev->next = node->next;
173 _tab[i] = node->next;
180 {
for(
const auto x: c)
remove(x); }
186 for(node_t *n = _tab[i.i]; n != i.node;
p = n, n = n->next);
188 _tab[i.i] = i.node->next;
190 p->next = i.node->next;
199 for(
int i = 0; i < _size; i++) {
200 if(
t._tab[i] !=
nullptr) {
201 node_t *q =
t._tab[i];
202 node_t *
p =
new(A::allocate(
sizeof(node_t))) node_t(q->data);
204 while(q->next !=
nullptr) {
206 p->next =
new(A::allocate(
sizeof(node_t))) node_t(q->data);
215 inline T *
get(
const T& key)
216 { node_t *node =
find(key);
return node ? &node->data : 0; }
219 int minEntry(
void)
const {
int m =
count(0);
for(
int i = 1; i < _size; i++) m =
min(m,
count(i));
return m; }
220 int maxEntry(
void)
const {
int m =
count(0);
for(
int i = 1; i < _size; i++) m =
max(m,
count(i));
return m; }
221 int zeroEntry(
void)
const {
int c = 0;
for(
int i = 0; i < _size; i++)
if(
count(i) == 0) c++;
return c; }
222 int size(
void)
const {
return _size; }
227 int count(
int i)
const {
int c = 0;
for(node_t *n = _tab[i]; n; n = n->next) c++;
return c; }
bool operator!=(const HashTable< T > &t) const
Definition: HashTable.h:149
Printable< T, M > p(const T &data, const M &man)
Definition: Output.h:302
void removeAll(const C &c)
Definition: HashTable.h:179
bool hasKey_const(const T &key) const
Definition: HashTable.h:109
Iter end() const
Definition: HashTable.h:142
self_t & operator-=(const T &x)
Definition: HashTable.h:182
self_t & operator+=(const T &x)
Definition: HashTable.h:161
void remove(const Iter &i)
Definition: HashTable.h:184
bool containsAll(const CC &c) const
Definition: HashTable.h:130
const T & max(const T &x, const T &y)
Definition: compare.h:108
~HashTable(void)
Definition: HashTable.h:96
bool contains_const(const T &x) const
Definition: HashTable.h:128
void addAll(const C &c)
Definition: HashTable.h:163
const H & hash() const
Definition: HashTable.h:98
HashTable(const self_t &h)
Definition: HashTable.h:94
Definition: HashTable.h:135
const A & allocator() const
Definition: HashTable.h:100
A & allocator()
Definition: HashTable.h:101
t::ptr allocate(t::size size) const
Definition: custom.h:31
void put(const T &data)
Definition: HashTable.h:114
const T & min(const T &x, const T &y)
Definition: compare.h:104
const T & item(void) const
Definition: HashTable.h:139
bool equals(const HashTable< T > &h) const
Definition: HashTable.h:144
const T * get(const T &key) const
Definition: HashTable.h:103
bool equals_const(const HashTable< T > &h) const
Definition: HashTable.h:146
Iter(const self_t &htab, bool end)
Definition: HashTable.h:138
void copy(const HashTable< T, H > &t)
Definition: HashTable.h:194
bool hasKey(const T &key) const
Definition: HashTable.h:107
void putAll(const CC &c)
Definition: HashTable.h:116
bool operator==(const HashTable< T > &t) const
Definition: HashTable.h:148
uint64 size
Definition: arch.h:35
Iter(const self_t &htab)
Definition: HashTable.h:137
const T * get_const(const T &key) const
Definition: HashTable.h:105
int count(void) const
Definition: HashTable.h:124
H & hash()
Definition: HashTable.h:99
void clear(void)
Definition: HashTable.h:152
void remove(const T &key)
Definition: HashTable.h:166
Definition: HashTable.h:32
bool contains(const T &x) const
Definition: HashTable.h:126
T * add(const T &data)
Definition: HashTable.h:159
bool exists(const T &key) const
Definition: HashTable.h:111
Iter begin() const
Definition: HashTable.h:141
static void clear(T *target, int size)
Definition: array.h:42
HashTable< T, H, A > self_t
Definition: HashTable.h:34
HashTable(int _size=211)
Definition: HashTable.h:92
self_t & operator=(const HashTable< T, H > &c)
Definition: HashTable.h:213
node_t * find(const T &key) const
Definition: HashTable.h:45
bool containsAll_const(const CC &c) const
Definition: HashTable.h:132
T * get(const T &key)
Definition: HashTable.h:215
bool exists_const(const T &key) const
Definition: HashTable.h:112
node_t * find_const(const T &key) const
Definition: HashTable.h:55
bool isEmpty(void) const
Definition: HashTable.h:121