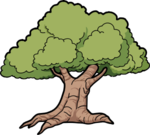 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_DATA_SLICE_H_
22 #define ELM_DATA_SLICE_H_
24 #include <elm/PreIterator.h>
32 typedef typename C::t
t;
34 inline Slice(): a(nullptr), f(0), c(0) { }
40 inline int count()
const {
return c; }
42 inline const t&
get(
int i)
const {
return (*a)[f + i]; }
44 inline t&
get(
int i) {
return (*a)[f + i]; }
50 inline bool ended()
const {
return i >=
s.c; }
51 inline const t&
item()
const {
return s[
i]; }
61 inline int indexOf(
const t& x,
int i = 0)
const
62 {
for(; i < c; i++)
if(x ==
get(i))
return i;
return -1; }
64 {
if(i == -1) i = c - 1;
for(; i >= 0; i--)
if(x ==
get(i))
break;
return i; }
78 {
for(
auto x: s)
if(!
contains(x))
return false;
return true; }
79 inline bool isEmpty()
const {
return c == 0; }
82 if(c != s.c)
return false;
83 for(
int i = 0; i < c; i++)
if(
get(i) != s.
get(i))
return false;
void next()
Definition: Slice.h:52
Slice< C > self_t
Definition: Slice.h:31
C::t t
Definition: Slice.h:32
Slice(C &array, int first, int count)
Definition: Slice.h:35
bool isEmpty() const
Definition: Slice.h:79
bool operator==(const self_t &s) const
Definition: Slice.h:86
Iter begin() const
Definition: Slice.h:72
bool ended() const
Definition: Slice.h:50
int count() const
Definition: Slice.h:40
const self_t & s
Definition: Slice.h:55
int lastIndex() const
Definition: Slice.h:39
MutIter end()
Definition: Slice.h:96
Iter end() const
Definition: Slice.h:73
int lastIndexOf(const t &x, int i=-1) const
Definition: Slice.h:63
t & operator[](int i)
Definition: Slice.h:45
const t & item() const
Definition: Slice.h:70
int indexOf(const t &x, int i=0) const
Definition: Slice.h:61
Slice< C > slice(C &a, int fst, int cnt)
Definition: Slice.h:104
int i
Definition: Slice.h:56
t & get(int i)
Definition: Slice.h:44
int length() const
Definition: Slice.h:60
MutIter begin()
Definition: Slice.h:95
t & item()
Definition: Slice.h:93
Slice()
Definition: Slice.h:34
const t & get(int i) const
Definition: Slice.h:42
bool contains(const t &x) const
Definition: Slice.h:75
const t & item() const
Definition: Slice.h:51
bool equals(const self_t &s) const
Definition: Slice.h:81
MutIter(self_t &slice, int idx=0)
Definition: Slice.h:92
C & array() const
Definition: Slice.h:37
bool operator!=(const self_t &s) const
Definition: Slice.h:87
bool equals(const BaseIter &ii) const
Definition: Slice.h:53
BaseIter(const self_t &slice, int idx=0)
Definition: Slice.h:49
const t & operator[](int i) const
Definition: Slice.h:43
int firstIndex() const
Definition: Slice.h:38
bool containsAll(const self_t &s) const
Definition: Slice.h:77