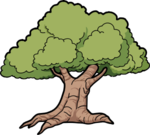 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
22 #ifndef ELM_UTIL_VERSION_H
23 #define ELM_UTIL_VERSION_H
25 #include <elm/assert.h>
41 inline Version(
const string& text) { *
this = text; }
47 inline int major(
void)
const;
48 inline int minor(
void)
const;
54 inline operator bool(
void)
const;
68 unsigned short _release;
71 io::Output&
operator<<(io::Output&
out,
const Version& version);
75 : _major(major), _minor(minor), _release(release) {
76 ASSERTP(
major >= 0,
"major number must be positive");
77 ASSERTP(
minor >= 0,
"minor number must be positive");
78 ASSERTP(
release >= 0,
"release number be positive");
82 : _major(version._major), _minor(version._minor), _release(version._release) {
86 return Version(_major, _minor, _release + 1);
90 return Version(_major, _minor + 1, 0);
94 return Version(_major + 1, 0, 0);
110 return _major != version._major || _minor <= version._minor;
114 int res = _major - version._major;
116 res = _minor - version._minor;
120 inline Version::operator
bool(
void)
const {
121 return _major || _minor || _release;
125 _major = version._major;
126 _minor = version._minor;
127 _release = version._release;
157 #endif // ELM_UTIL_VERSION_H
bool operator==(const Version &version) const
Definition: Version.h:131
Version nextMinor(void) const
Definition: Version.h:89
typename type_info< T >::out_t out
Definition: type_info.h:284
Version nextMajor(void) const
Definition: Version.h:93
int compare(const Version &version) const
Definition: Version.h:113
bool operator<(const Version &version) const
Definition: Version.h:147
AutoString & operator<<(CString str, const T &value)
Definition: AutoString.h:75
bool operator<=(const Version &version) const
Definition: Version.h:151
static const Version ZERO
Definition: Version.h:34
Version & operator=(const char *text)
Definition: Version.h:56
int minor(void) const
Definition: Version.h:101
String string
Definition: String.h:149
bool operator>(const Version &version) const
Definition: Version.h:139
int release(void) const
Definition: Version.h:105
Version(const string &text)
Definition: Version.h:41
Version(int major=0, int minor=0, int release=0)
Definition: Version.h:74
int major(void) const
Definition: Version.h:97
Version nextRelease(void) const
Definition: Version.h:85
bool accepts(const Version &version) const
Definition: Version.h:109
Version(const char *text)
Definition: Version.h:39
bool operator>=(const Version &version) const
Definition: Version.h:143
Version(const cstring text)
Definition: Version.h:40
Version & operator=(const cstring text)
Definition: Version.h:57
bool operator!=(const Version &version) const
Definition: Version.h:135
Version & operator=(const Version &version)
Definition: Version.h:124