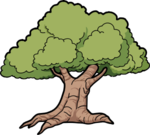 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_SYS_PATH_H
22 #define ELM_SYS_PATH_H
24 #include <elm/io/InStream.h>
25 #include <elm/io/Output.h>
26 #include <elm/PreIterator.h>
27 #include <elm/string.h>
28 #include <elm/sys/SystemException.h>
30 namespace elm {
namespace sys {
35 # if defined(__WIN32) || defined(__WIN64)
48 inline Path(
const char *path): buf(path) { }
51 inline Path(
const Path& path): buf(path.buf) { }
72 inline bool equals(
Path& path)
const {
return buf == path.buf; }
85 bool isDir(
void)
const;
107 inline operator bool (
void)
const {
return buf; }
114 inline PathIter(
string paths): s(paths),
p(0), n(-1) { look(); }
120 inline PathIter(
string paths,
int p): s(paths),
p(
p), n(-1) { look(); }
144 inline bool ended(
void)
const {
return _dir ==
nullptr; }
147 inline bool equals(
const DirIter& i)
const {
return _dir == i._dir && _cur == i._cur; }
178 int nextSeparator(
int start = 0)
const;
179 int lastSeparator(
void)
const;
192 #endif // ELM_SYS_PATH_H
bool operator!=(const DirIter &i) const
Definition: Path.h:155
bool equals(const PathIter &i) const
Definition: Path.h:118
bool isFile(void) const
Definition: system_Path.cpp:559
bool ended(void) const
Definition: Path.h:115
bool equals(Path &path) const
Definition: Path.h:72
String extension(void) const
Definition: system_Path.cpp:495
typename type_info< T >::out_t out
Definition: type_info.h:284
Path & operator=(const char *str)
Definition: Path.h:98
Path item(void) const
Definition: Path.h:116
Printable< T, M > p(const T &data, const M &man)
Definition: Output.h:302
static const string BACK_PATH
Definition: Path.h:44
static void setCurrent(Path &path)
Definition: system_Path.cpp:200
DirReader readDir(void) const
Definition: Path.h:171
Path absolute(void) const
Definition: system_Path.cpp:186
void makeDirs(void) const
Definition: system_Path.cpp:687
DirIter(void)
Definition: Path.h:141
static const char SEPARATOR
Definition: Path.h:40
bool contains(const Path &path) const
Definition: Path.h:175
DirIter begin(void)
Definition: Path.h:165
Path(CString path)
Definition: Path.h:49
Path setExt(cstring ext) const
Definition: Path.h:58
bool isRelative(void) const
Definition: system_Path.cpp:294
io::OutStream * append(void)
Definition: system_Path.cpp:659
const String & toString(void) const
Definition: Path.h:63
DirReader(const Path &p)
Definition: Path.h:164
IntFormat base(int base, IntFormat fmt)
Definition: Output.h:256
bool isAbsolute(void) const
Definition: system_Path.cpp:274
Path(const char *path)
Definition: Path.h:48
static Path current(void)
Definition: system_Path.cpp:349
static PathSplit splitPaths(string paths)
Definition: Path.h:134
cstring operator*() const
Definition: Path.h:151
CString cstring
Definition: CString.h:62
bool equals(const DirIter &i) const
Definition: Path.h:147
Path operator/(const Path &path) const
Definition: Path.h:105
Path & operator=(const String &str)
Definition: Path.h:100
bool subPathOf(const Path &path) const
Definition: Path.h:73
bool prefixedBy(const Path &path) const
Definition: Path.h:76
cstring t
Definition: Path.h:139
void makeDir(void)
Definition: system_Path.cpp:626
Path canonical(void) const
Definition: system_Path.cpp:134
bool operator==(Path path) const
Definition: Path.h:103
cstring item(void) const
Definition: Path.h:145
Path & operator=(CString str)
Definition: Path.h:99
bool isDir(void) const
Definition: system_Path.cpp:572
Path relativeTo(Path base) const
Definition: system_Path.cpp:114
Path parent(void) const
Definition: system_Path.cpp:228
Path(void)
Definition: Path.h:47
int length(void) const
Definition: String.h:75
bool isPrefixOf(const Path &path) const
Definition: Path.h:74
bool operator==(const DirIter &i) const
Definition: Path.h:154
const char * asSysString() const
Definition: Path.h:80
sys::Path dirPart(void) const
Definition: system_Path.cpp:261
bool isWritable(void) const
Definition: system_Path.cpp:595
bool isEmpty(void) const
Definition: Path.h:68
const char * chars(void) const
Definition: CString.h:27
io::InStream * read(void)
Definition: system_Path.cpp:636
static bool isSeparator(char c)
Definition: Path.h:42
void remove(void)
Definition: system_Path.cpp:617
Path(const Path &path)
Definition: Path.h:51
PathIter end(void) const
Definition: Path.h:130
bool ended(void) const
Definition: Path.h:144
bool isExecutable(void) const
Definition: system_Path.cpp:604
String substring(int _off) const
Definition: String.h:96
PathIter begin(void) const
Definition: Path.h:129
Definition: OutStream.h:30
elm::sys::Path Path
Definition: Path.h:188
Path setExtension(CString new_extension) const
Definition: system_Path.cpp:510
Path withoutExt(void) const
Definition: system_Path.cpp:535
void next(void)
Definition: Path.h:117
DirIter & operator++()
Definition: Path.h:152
PathIter(void)
Definition: Path.h:113
io::Output & operator<<(io::Output &out, const Path &path)
Definition: Path.h:183
io::OutStream * write(void)
Definition: system_Path.cpp:647
DirIter operator++(int)
Definition: Path.h:153
static const char PATH_SEPARATOR
Definition: Path.h:41
PathSplit(string p)
Definition: Path.h:128
PathIter(string paths)
Definition: Path.h:114
void next(void)
Definition: system_Path.cpp:716
Path basePart(void) const
Definition: system_Path.cpp:481
bool startsWith(const char *str) const
Definition: String.h:112
string str(const char *s)
Definition: String.h:150
DirIter end(void)
Definition: Path.h:166
static Path temp(void)
Definition: system_Path.cpp:385
CString toCString(void) const
Definition: String.h:90
bool isEmpty(void) const
Definition: String.h:87
Path & operator=(const Path &path)
Definition: Path.h:101
bool operator!=(Path path) const
Definition: Path.h:104
String namePart(void) const
Definition: system_Path.cpp:248
static Path home(void)
Definition: system_Path.cpp:366
bool isReadable(void) const
Definition: system_Path.cpp:585
bool exists(void) const
Definition: system_Path.cpp:549
Definition: InStream.h:29
Path(const String &path)
Definition: Path.h:50
bool isHomeRelative(void) const
Definition: system_Path.cpp:303