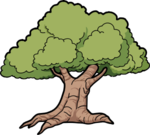 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef ELM_UTIL_LOCKPTR_H
22 #define ELM_UTIL_LOCKPTR_H
24 #include <elm/assert.h>
33 inline void lock(
void) { u++; }
35 inline int usage(
void)
const {
return u; }
42 inline LockPtr(T *
p = 0): ptr(
p ?
p : null_lock()) { ptr->lock(); }
48 { unlock(); ptr = lock.ptr; ptr->lock();
return *
this;}
50 { unlock(); ptr =
p ?
p : null_lock(); ptr->lock();
return *
this; }
53 { ASSERTP(!
isNull(),
"accessing null pointer");
return (T *)ptr; }
55 { ASSERTP(!
isNull(),
"accessing null pointer");
return *(T *)ptr; }
57 {
return isNull() ? 0 : (T *)ptr; }
60 {
return ptr == null_lock(); }
64 {
return ptr == ap.ptr; }
66 {
return ptr != ap.ptr; }
68 {
return ptr > ap.ptr; }
70 {
return ptr >= ap.ptr; }
72 {
return ptr < ap.ptr; }
74 {
return ptr <= ap.ptr; }
77 void unlock(
void) { ptr->unlock();
if(!ptr->usage()) {
delete ptr; ptr = null_lock(); } }
79 static T *null_lock(
void) {
static Lock n(1);
return (T *)&n; }
84 #endif // ELM_UTIL_AUTOPTR_H
T * operator->(void) const
Definition: LockPtr.h:52
Printable< T, M > p(const T &data, const M &man)
Definition: Output.h:302
T & operator*(void) const
Definition: LockPtr.h:54
T * operator&(void) const
Definition: LockPtr.h:56
int usage(void) const
Definition: LockPtr.h:35
bool operator>(const LockPtr< T > &ap) const
Definition: LockPtr.h:67
void unlock(void)
Definition: LockPtr.h:34
bool isNull(void) const
Definition: LockPtr.h:59
Lock(int usage=0)
Definition: LockPtr.h:32
void lock(void)
Definition: LockPtr.h:33
LockPtr(T *p=0)
Definition: LockPtr.h:42
LockPtr(const LockPtr &l)
Definition: LockPtr.h:43
bool operator>=(const LockPtr< T > &ap) const
Definition: LockPtr.h:69
bool operator<=(const LockPtr< T > &ap) const
Definition: LockPtr.h:73
LockPtr & operator=(const LockPtr &lock)
Definition: LockPtr.h:47
bool operator!=(const LockPtr< T > &ap) const
Definition: LockPtr.h:65
~LockPtr(void)
Definition: LockPtr.h:44
LockPtr & operator=(T *p)
Definition: LockPtr.h:49
bool operator==(const LockPtr< T > &ap) const
Definition: LockPtr.h:63
bool operator<(const LockPtr< T > &ap) const
Definition: LockPtr.h:71