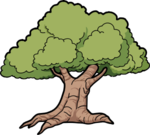 |
Elm
2
ELM is a library providing generic data structures, OS-independent interface, plugins and XML.
|
21 #ifndef INCLUDE_ELM_XOM_DTD_H_
22 #define INCLUDE_ELM_XOM_DTD_H_
24 #include <elm/data/HashMap.h>
25 #include <elm/data/List.h>
26 #include <elm/io/InStream.h>
29 namespace elm {
namespace dtd {
54 {
return asElement()->getAttributeValue(name); }
58 { ASSERT(!stack.isEmpty());
return static_cast<xom::Element *
>(stack.top().node); }
61 inline void raise(
const string& msg)
const {
throw Exception(asElement(), msg); }
62 inline void fail(
const string& msg)
const {
throw Exception(_last_error, msg); }
65 inline bool ended()
const {
return cur.node ==
nullptr; }
67 inline void next(
void) { cur.i++; setNode(); }
74 void end(
bool success);
89 : attr(a), object(o), xelt(xe) { }
90 AbstractAttribute *attr;
115 virtual void begin(
Element& element);
116 virtual void end(
Element& element);
117 virtual void backtrack(
Element& element);
118 virtual void *getRef(
Element& element);
141 inline bool isSet()
const {
return _set; }
147 bool parse(
Parser& parser);
149 virtual void reset();
150 virtual void postprocess(
Parser& parser);
164 virtual void reset() = 0;
168 virtual bool parse(
Parser& parser) = 0;
191 void reset()
override;
192 bool parse(
Parser& parser)
override;
204 void reset()
override;
205 bool parse(
Parser& parser)
override;
215 bool parse(
Parser& parser)
override;
216 void reset()
override;
225 bool parse(
Parser& parser)
override;
226 void reset()
override;
236 bool parse(
Parser& parser)
override;
237 void reset()
override;
278 void reset()
override;
279 void postprocess(
Parser& parser)
override;
291 inline bool done()
const {
return _done; }
305 { parser.
addPost(
this);
return true; }
307 parser.
raise(
_ <<
"undefined reference \"" <<
value <<
"\" in " << name());
static const t::uint32 FORWARD
Definition: dtd.h:132
Definition: MessageException.h:30
Content & operator+(Content &c1, Content &c2)
Definition: xom_dtd.cpp:1200
bool operator==(const CString &s1, const CString &s2)
Definition: string.h:35
void * node
Definition: Node.h:60
bool hasID(xom::String id) const
Definition: dtd.h:76
void next(void)
Definition: dtd.h:67
xom::String name() const
Definition: dtd.h:179
bool doParse(Content &content, Parser &parser)
Definition: dtd.h:167
static const t::uint32 CROP
Definition: dtd.h:46
bool backtrack(mark_t m)
Definition: dtd.h:71
const T & operator*() const
Definition: dtd.h:248
mut< T > ref(var< T > &x)
Definition: type_info.h:289
virtual String message(void)
Definition: utility.cpp:79
bool ended() const
Definition: dtd.h:65
xom::String operator*() const
Definition: dtd.h:275
bool process(Parser &parser, xom::String value) override
Definition: dtd.h:250
Content & ANY
Definition: xom_dtd.cpp:1070
void reset() override
Definition: dtd.h:252
Element & element() const
Definition: dtd.h:137
xom::Node * current(void) const
Definition: dtd.h:66
const t::uint32 STRICT
Definition: dtd.h:333
bool done() const
Definition: dtd.h:291
Definition: Exception.h:29
const t::uint32 CROP
Definition: dtd.h:336
void recordError()
Definition: dtd.h:63
Option< xom::String > get(xom::String name) const
Definition: dtd.h:53
bool process(Parser &parser, xom::String value) override
Definition: dtd.h:265
xom::Element * parent() const
Definition: dtd.h:57
static const t::uint32 REQUIRED
Definition: dtd.h:130
mark_t mark(void)
Definition: dtd.h:70
Content & operator*(Content &c)
Definition: xom_dtd.cpp:1185
static const t::uint32 STRICT
Definition: dtd.h:131
xom::String id() const
Definition: dtd.h:292
bool operator==(const AbstractAttribute &a) const
Definition: dtd.h:143
Content & ignored
Definition: xom_dtd.cpp:714
virtual void reset()
Definition: xom_dtd.cpp:631
Content & PCDATA
Definition: xom_dtd.cpp:1047
Attribute(Element &element, xom::String name, const T &init, t::uint32 flags=0)
Definition: dtd.h:246
Content & operator,(Content &c1, Content &c2)
Definition: xom_dtd.cpp:1218
Element & element(void) const
Definition: dtd.h:52
typename type_info< T >::in_t in
Definition: type_info.h:283
void addPost(AbstractAttribute *att)
Definition: dtd.h:78
const t::uint32 FORWARD
Definition: dtd.h:335
AutoStringStartup & _
Definition: debug_CrashHandler.cpp:232
Content & operator&(Content &c1, Content &c2)
Definition: xom_dtd.cpp:1226
bool doesCrop() const
Definition: dtd.h:50
xom::String CDATA
Definition: dtd.h:324
bool isRequired() const
Definition: dtd.h:138
Content & EMPTY
Definition: xom_dtd.cpp:694
virtual bool parse(Parser &parser)=0
Content & content() const
Definition: dtd.h:180
xom::String name() const
Definition: dtd.h:135
Definition: Document.h:20
Factory & factory(void) const
Definition: dtd.h:51
xom::Element * asElement() const
Definition: dtd.h:55
RefAttribute(Element &element, xom::String name, t::uint32 flags=0)
Definition: dtd.h:288
unsigned int uint32
Definition: arch.h:31
bool isSet() const
Definition: dtd.h:141
void raise(const string &msg) const
Definition: dtd.h:61
void postprocess(Parser &parser) override
Definition: dtd.h:315
bool isForward() const
Definition: dtd.h:140
void recordPatch(xom::String id, AbstractAttribute &attr)
Definition: xom_dtd.cpp:360
int mark_t
Definition: dtd.h:69
bool operator!=(const AbstractAttribute &a) const
Definition: dtd.h:144
void reset() override
Definition: dtd.h:312
void fail(const string &msg) const
Definition: dtd.h:62
bool operator!=(const Element &element) const
Definition: dtd.h:183
const t::uint32 REQUIRED
Definition: dtd.h:334
Content & operator!(Content &c)
Definition: xom_dtd.cpp:1192
Attribute(Element &element, xom::String name, const xom::String &init, t::uint32 flags=0)
Definition: dtd.h:261
Content & operator|(Content &c1, Content &c2)
Definition: xom_dtd.cpp:1208
void * getID(xom::String id) const
Definition: dtd.h:77
xom::String operator*() const
Definition: dtd.h:263
const Vector< AbstractAttribute * > & attributes() const
Definition: dtd.h:181
void reset() override
Definition: dtd.h:266
bool process(Parser &parser, xom::String value) override
Definition: dtd.h:294
bool operator==(const Element &element) const
Definition: dtd.h:182
bool isStrict() const
Definition: dtd.h:139
Definition: InStream.h:29
T * operator*() const
Definition: dtd.h:290